반응형
🚩[예제 1] 한 줄 옆으로 출력(print), 한 줄 띄우고 출력(println)
1
2
3
4
5
6
7
8
|
public class Main {
public static void main(String[] args) {
System.out.print("Hello.");
System.out.print("Nice to meet you.\n");
System.out.println("Good bye.");
System.out.println("See you later.");
}
}
|
cs |
🏴 [유제 1] 다음과 같은 출력이 나오는 프로그램을 만드세요.
Hello World! I am a Java program. Nice to meet you.
더보기
public class Main {
public static void main(String[] args) {
System.out.println("Hello World!");
System.out.print("I am a Java program.");
System.out.print("Nice to meet you.");
}
}
🚩[예제 2] 문자 + 문자
- + 연산자는 숫자뿐만이 아니라 문자열과 문자열을 결합(또는 문자열과 숫자)할 때도 사용 가능 합니다.
1
2
3
4
5
6
7
8
9
10
11
|
public class Main {
public static void main(String[] args) {
System.out.println("Hello~" + "Tom"); // Hello~Tom
System.out.println("1" + "1"); // 11
System.out.println('1' + '1'); // 98 (49+49)
System.out.println(1+1); // 2
System.out.println("A" + "B"); // AB
System.out.println('A' + 'B'); // 131 (65+66)
System.out.print("Hello" + 123); // Hello123
}
}
|
cs |
더보기
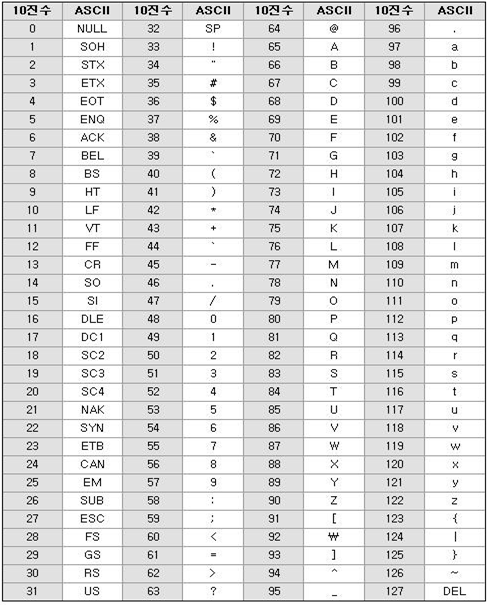
아스키코드 표
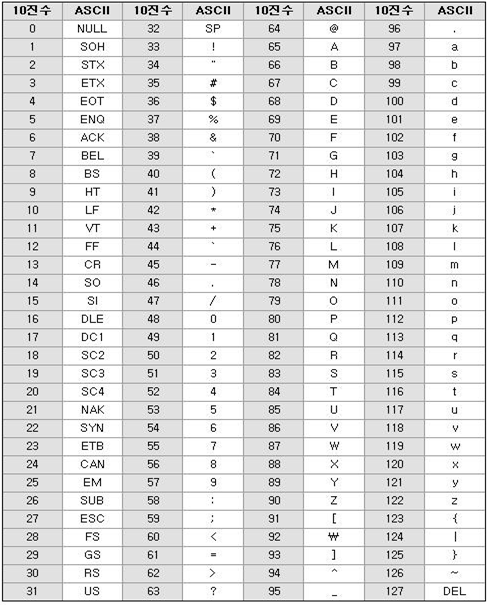
🏴 [유제 2] 다음의 지시사항을 코딩해 보세요.
▶첫번째 줄에 "Machine"과 "Learning"을 결합해서 출력합니다.
▶두번째 줄에 숫자 5+10을 출력합니다.
▶세번째 줄에 문자열 "12" + "15"를 출력합니다.
▶네번째 줄에 "Hello~"와 "World"를 결합해서 출력합니다.
▶다섯번째 줄에 "apple"과 숫자 100을 결합해서 출력합니다.
더보기
public class Main {
public static void main(String[] args) {
System.out.println("Machine" + "Learning");// MachineLearning
System.out.println(5+10); // 15
System.out.println("12" + "15");// 1215
System.out.println("Hello~"+"World"); // Hello~World
System.out.print("apple" + 100); // apple100
}
}
🚩[예제 3] 변수
- 변수(variable)란 데이터를 저장하는 공간입니다.
- 데이터는 숫자, 문자들 입니다.
- int a = 1 에서 처럼, int는 자료형(data type), a는 변수이름, =은 할당(저장)하기, 1은 변수의 초기값 입니다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
public class Main {
public static void main(String[] args) {
byte a = -128; // what if -129 ?
short b = 32767; // what if 32768 ?
double c = 3.14;
boolean d = false;
char e1 = 'a';
char e2 = 97;
System.out.println("byte a: " + a); // -128
System.out.println("int b: " + b); // 32767
System.out.println("float c: " + c); // 3.14
System.out.println("boolean d: " + d); // false
System.out.println("char e1: " + e1); // a
System.out.println("char e2: " + e2); // a
}
}
|
cs |
🏴 [유제 3] 다음의 지시사항을 코딩해 보세요.
▶ 정수형(int)변수 x에 1000을 저장하고, 화면에 아래와 같이 출력합니다.
x is 1000
▶ 정수형(short) 변수 y에 0을 저장하고, y에 1을 더해서 화면에 아래와 같이 출력합니다.
y is 0. y + 1 is 1.
▶ 실수형(double)변수 z에 아무 실수값을 저장하고 화면에 z를 출력해 보세요.
▶ 문자형(char)변수 k에 알파벳 P를 저장하고, 화면에 변수 k를 출력해 보세요.
더보기
public class Main {
public static void main(String[] args) {
int x = 1000;
short y = 0;
double z = 3.1415;
char k = 'P';
System.out.println("x is " + x);
System.out.println("y is 0.");
System.out.println("y + 1 is " + (y + 1)); // y+1:01, (y+1):1
System.out.println(z);
System.out.println(k);
}
}
🚩[예제 4] 빛이 1년동안 갈 수 있는 거리
1
2
3
4
5
6
7
8
9
10
|
public class Main {
public static void main(String[] args) {
long lightSpeed = 300000; // km/s
long timeOfyear = 365 * 24 * 60 * 60;
long distance;
distance = lightSpeed * timeOfyear;
System.out.println("빛이 1년 동안 가는 거리:" + distance + "km");
}
}
|
cs |
🏴 [유제 4] 반지름이 4.0인 원의 넓이를 계산하는 프로그램을 만드시오.
- 변수의 자료형은 double로 하세요.
- 원 넓이 공식: 3.14 x 반지름 x 반지름
더보기
public class Main {
public static void main(String[] args) {
double radius = 4.0;
double area;
area = 3.14 * radius * radius;
System.out.println("원의 넓이:" + area);
}
}
🚩[예제 5] 특수문자
1
2
3
4
5
6
7
8
|
public class Main {
public static void main(String[] args) {
System.out.print("Good Morning\n"); // Good Morning
System.out.println("\"Hello World\"");// "Hello World"
System.out.println("\tNice to meet you."); // nice to meet you
System.out.println("c:\\myFolder\\paint.png"); // c:\myFolder\paint.png
}
}
|
cs |
🏴 [유제 5] 아래와 같이 출력을 해보세요.
D:\windows\user\document
'The Times'
"Lake City"
🚩[예제 6] 논리형
- boolean형은 true 또는 false 값만 가질 수 있다.
1
2
3
4
5
6
7
8
9
10
11
12
13
|
public class Main {
public static void main(String[] args) {
int num = 100;
boolean a, b, c;
a = 2 > 1;
b = 1 != 1;
c = num > 1000;
System.out.println("a: " + a); // true
System.out.println("b: " + b); // false
System.out.println("c: " + c); // false
}
}
|
cs |
🏴 [유제 6] 다음의 실행했을 때, 변수 a, b, c에는 무엇이 저장될까요?
boolean a, b, c;
a = 100 <= 1;
b = 5 == 5;
c = -1*5 > 1000;
더보기
a: false
b: true
c: false
🚩[예제 6] 변수 초기화를 안한다면?
- 변수를 초기화 하지 않고 사용하게 되면 오류가 발생한다.
1
2
3
4
5
6
7
|
public class Main {
public static void main(String[] args) {
int a;
System.out.println(a);
//error: variable a might not have been initialized
}
}
|
cs |
🚩[예제 7] 변수이름 규칙
- 변수이름은 유니코드 문자(영어, 한글 등등...)와 숫자의 조합
- 변수이름의 첫 글자는 유니코드 문자, _로 시작(숫자로 시작X)
- 변수이름의 두 번째 글자부터는 문자,숫자,_,$ 등이 가능하다.
- 대문자와 소문자는 구별된다.
- 변수이름으로 키워드(if, for 등..)를 사용할 수 없다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
public class Main {
public static void main(String[] args) {
// OK
int age;
long myAge;
int _count;
long $money;
int 한글변수;
int Apple14;
// NO
int 1stNumber;
double if;
int #ofBook;
}
}
|
cs |
🏴 [유제 7] 아래의 변수이름 중 오류가 나는 것은?
int for;
double 45years;
int good morning;
int my_number;
long what$is;
더보기
int for; // 오류
double 45years; // 오류
int good morning; // 오류
🚩[예제 8] 상수
- 상수란 항상 "상"자를 사용하여, 변하지 않고 항상 고정된 값을 담는 변수를 의미합니다.
- 자바에서 상수를 만들 때는 final 이라는 명령어를 제일 왼쪽에 붙여줍니다.
1
2
3
4
5
6
7
8
9
10
|
public class Main {
public static void main(String[] args) {
final double PI = 3.14; // 상수(constant)
int radius = 5;
double area = 0;
area = PI * radius * radius;
System.out.print("area = " + area);
}
}
|
cs |
🏴 [유제 8] 아래의 값 중, 빛의 속도를 상수(final)로 만들어 지시사항대로 출력해 보세요.
-빛의 속도: 300,000 (km/sec)
-시간 = 거리 / 속도
-지시사항: 거리 600,000 (km)를 빛의 속도로 날아가면 걸리는 시간을 출력하는 프로그램을 만들어 보세요.
더보기
public class Main {
public static void main(String[] args) {
final double speedOfLight = 300000; // 빛의속도
int distance = 600000;
double amountOfTime = 0;
amountOfTime = distance / speedOfLight;
System.out.print("amountOfTime = " + amountOfTime);
}
}
728x90
반응형
'자바(Java) > 자바기초' 카테고리의 다른 글
[자바기초] 2차원 배열(2D Array) (0) | 2024.03.03 |
---|---|
[자바기초]1차원 배열(array) (0) | 2024.03.01 |
[자바기초.006]코드업100제_기초출력풀이_1001~1007 (0) | 2022.09.12 |
[자바기초.003]문자열,입력 (0) | 2022.07.19 |
[자바기초.002]연산자,형변환,입력 (0) | 2022.07.18 |
댓글