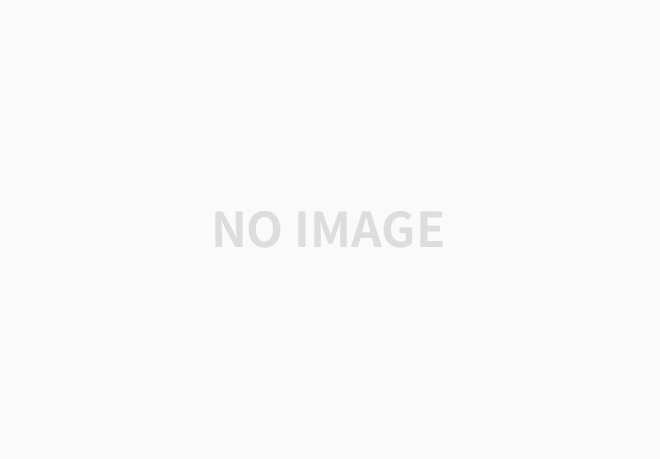
[자바기초.017] 상속 계층(Inheritance Hierarchies)
[1] 상속 계층
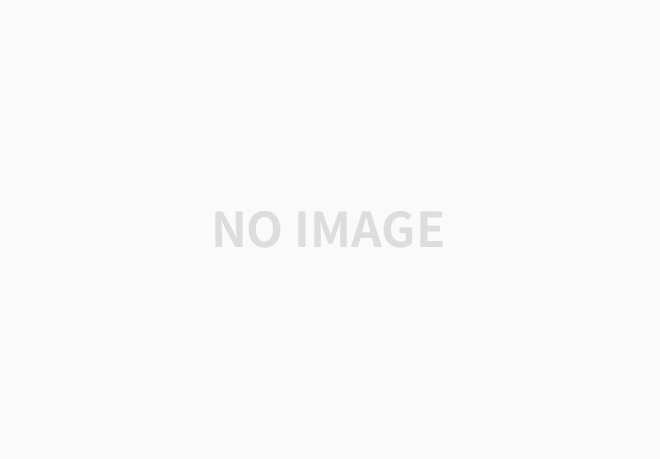
- 하나의 superclass에서 여러 개의 subclass가 상속을 받는 관계일 때, 상속 계층(inheritance hierarchy)로 표시하면 코딩하는 데에 도움이 될 수 있다.
- 위 그림을 예로 들면, Square is-a Rectangle 이어서 Square 클래스는 Rectangle 클래스의 subclass 입니다.
- 그리고 Rectangle is-a Shape 이어서 Rectangle 클래스는 Shape 클래스의 subclass 입니다.
- 자바에서 Object 클래스는 계층(hierarchy)의 가장 꼭대기에 있는 클래스입니다.
- Object 클래스는 모든 자바 클래스의 최고 조상 클래스가 됩니다. 따라서 자바의 모든 클래스는 Object 클래스의 모든 메소드를 바로 사용할 수 있습니다.
- 이러한 Object 클래스는 필드를 가지지 않으며, 총 11개의 메소드만(equals, toString,....)으로 구성되어 있습니다.
- 상속 계층으로 클래스를 표시하는 이유는, subclass로 만든 object(instance)는 superclass의 필드와 메소드를 상속받아 쓰므로 새롭게 필드와 메소드를 중복으로 선언할 필요가 없습니다. 따라서 어떤 클래스와 상속관계가 있는지 그림으로 잘 표현해두면 superclass의 필드와 메소드를 적절하게 잘 이용할 수 있습니다.
[퀴즈1] What variables and methods might be inherited from the superclass Shape in the inheritance hierarchy above?
[퀴즈2] Can you make a 3 level inheritance hierarchy for living things on Earth?
[2] 부모 클래스 참조(Superclass references)
- superclass 참조변수(reference variable)은 자기 자신의 클래스의 object를 가질 수도 있지만, subclass의 object도 가질 수 있습니다.(가진다 => 주소값을 가지고 있어 그곳을 가리키다 => 참조한다 =>)
- 예를 들어, Shape 클래스의 참조 변수 Shape sh는 Rectangle이나 Square 클래스의 object를 참조할 수 있습니다. 이런 특징을 polymorphism(다형성) 이라고 합니다.
1 2 3 4 | // The variables declared of type Shape can hold objects of its subclasses Shape s1 = new Shape(); Shape s2 = new Rectangle(); Shape s3 = new Square(); | cs |
- 하지만, 위 코드의 반대로 코딩하면 에러가 납니다.
- 즉, subclass의 참조변수에 superclass의 object를 넣을 수는 없습니다.
- 예를 들어, Square reference variable에 Shape object를 넣을 수는 없는데, 그 이유는 "not all Shapes are Squares"이기 때문입니다.
- 아래 코드는 에러가 발생하는 데, 그 이유는 "Shape cannot be converted to Square"이기 때문입니다.
- (although you could use a type-cast to get it to be a (Square)).
1 2 3 4 | // A subclass variable cannot hold the superclass object! // A Square is-a Shape, but not all Shapes are Squares. Square q = new Shape(); // ERROR!! | cs |
[퀴즈3] A class Student inherits from the superclass Person. Which of the following assignment statements will give a compiler error?
A. Person p = new Person();
B. Person p = new Student();
C. Student s = new Student();
D. Student s = new Person();
[3] superclass method parameters
Inheritance hierarchy의 또 다른 장점으로는, superclass 타입의 파라미터를 메소드에 다양하게 사용할 수 있다는 점읻.
예를 들어, print(Shape v) 메소드의 파라미터인 Shape v에는 여러 타입의 subclass인 Rectangle, Squares,....가 들어갈 수 있다.
1 2 3 4 5 | // This will work with all Shape subclasses (Squares, Rectangles, etc.) too public void print(Shape s) { ... } | cs |
[예제1] 아래 코드에서 superclass method parameter의 다양한 쓰임새에 대한 장점을 이해해 보자.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 | class Person { private String name; public Person(String name) { this.name = name; } @Override // override from superclass(Object Class) public String toString() { return name; } } class Student extends Person { private int id; public Student(String name, int id) { super(name); this.id = id; } @Override // override from superclass public String toString() { return super.toString() + " " + id; } } public class Main { // This will implicitly call the toString() method of object p public void print(Person p) { System.out.println(p); // => object를 print하면 클래스명@hashcode값을 // 문자열로 출력할 toString 메소드가 자동호출됨. } public static void main(String[] args) { Person p = new Person("Sila"); Student s = new Student("Tully", 1001); Main m = new Main(); m.print(p); // call print with a Person m.print(s); // call print with a Student } } | cs |
[실행결과]
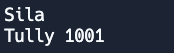
- Which toString() method is called above?
- What would happen if you commented out the Student toString() method? Which one would be called now?
- toString()함수를 주석처리해서 실행되지 않게 하여 print()가 그대로 실행하게 놔두면 아래와 같은 실행결과가 나옵니다.
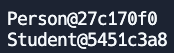
[참고]
PrintStream 클래스의 출력 관련 메소드(print, println 등)를 통해 객체를 출력하도록 명령하면 자바 컴파일러가 내부적으로 toString() 메소드를 호출한다.
[4] Superclass Arrays and ArrayLists
Inheritance hierarchies의 또다른 장점으로는, 배열(array)과 리스트(arrayList)를 superclass type과 함께 사용할 때, 여러개의 subclass type을 배열과 리스트 안에 저장할 수 있다는 것입니다. 아래의 예시 코드를 보세요.
1 2 3 4 5 6 7 8 | // This shape array can hold the subclass objects too Shape[] shapeArray = { new Rectangle(), new Square(), new Shape() }; // The shape ArrayList can add subclass objects too ArrayList<Shape> shapeList = new ArrayList<Shape>(); shapeList.add(new Shape()); shapeList.add(new Rectangle()); shapeList.add(new Square()); | cs |
[예제2]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | import java.util.*; class Pet { private String name; private String type; public Pet(String n, String t) { name = n; type = t; } public String toString() { return name + " is a " + type; } } class Dog extends Pet { public Dog(String n) { super(n, "dog"); } } public class Main { public static void main(String[] args) { ArrayList<Pet> petList = new ArrayList<Pet>(); petList.add(new Pet("Sammy", "hamster")); petList.add(new Dog("Fido")); // This loop will work for all subclasses of Pet for (Pet p : petList) { System.out.println(p); } } } | cs |
[실행결과]
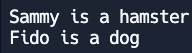
[유제2] 영어로 적힌 조건을 아래의 코드에 추가해서 완성하세요.
<조건>
1.Look at the Dog class and add a similar Cat class that extends Pet.(Don’t make the Cat class public because there can only be 1 public class in a file.)
2.Back to the main method and add some Cat objects to the ArrayList too. Does the petList work with Cats too?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 | import java.util.*; class Pet { private String name; private String type; public Pet(String n, String t) { name = n; type = t; } public String toString() { return name + " is a " + type; } } class Dog extends Pet { public Dog(String n) { super(n, "dog"); } } // [유제2] /* <조건> 1.Look at the Dog class and add a similar Cat class that extends Pet. 2.Back to the main method and add some Cat objects to the ArrayList too. Does the petList work with Cats too? */ public class Main { public static void main(String[] args) { ArrayList<Pet> petList = new ArrayList<Pet>(); petList.add(new Pet("Sammy", "hamster")); petList.add(new Dog("Fido")); // This loop will work for all subclasses of Pet for (Pet p : petList) { System.out.println(p); } } } | cs |
[유제3] 아래 문제의 정답을 고르세요.
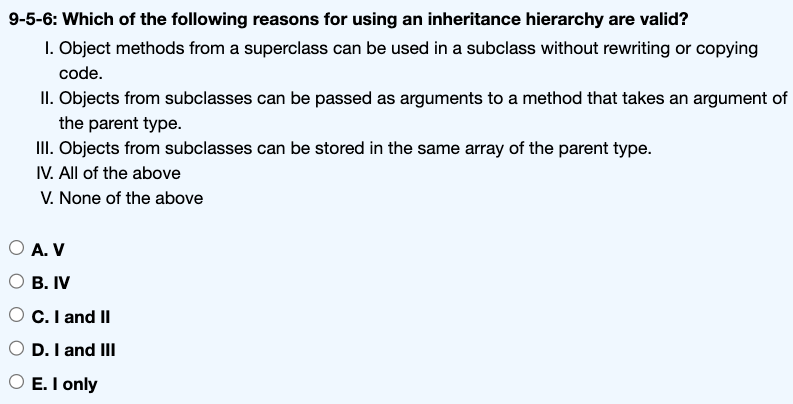
[퀴즈와 유제 정답은 아래 "더보기" 클릭]
[퀴즈1 정답]
length, area, .....
[퀴즈2 정답]
Animal-Person-Student
[퀴즈3 정답]
D
(This is not allowed because a Person is not always a Student.)
[유제2 정답]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 | import java.util.*; class Pet { private String name; private String type; public Pet(String n, String t) { name = n; type = t; } public String toString() { return name + " is a " + type; } } class Dog extends Pet { public Dog(String n) { super(n, "dog"); } } // [유제2] /* <조건> 1.Look at the Dog class and add a similar Cat class that extends Pet. 2.Back to the main method and add some Cat objects to the ArrayList too. Does the petList work with Cats too? */ class Cat extends Pet { public Cat(String n) { super(n, "cat"); } } public class Main { public static void main(String[] args) { ArrayList<Pet> petList = new ArrayList<Pet>(); petList.add(new Pet("Sammy", "hamster")); petList.add(new Dog("Fido")); petList.add(new Cat("Kitty")); // This loop will work for all subclasses of Pet for (Pet p : petList) { System.out.println(p); } } } | cs |
[유제3 정답]
B.IV
(All of these are valid reasons to use an inheritance hierarchy.)
'자바(Java) > 자바기초' 카테고리의 다른 글
[자바기초.019] Object Superclass (0) | 2024.03.17 |
---|---|
[자바기초.018] 다형성(Polymorphism) (0) | 2024.03.17 |
[자바기초.017] super 키워드 (0) | 2024.03.15 |
[자바기초.015] 오버라이딩(Overriding) (0) | 2024.03.15 |
[자바기초.014] is-a & has-a 관계 (1) | 2024.03.14 |
댓글