[자바기초] List & ArrayList
[1] List 란?
- List는 자바에서 제공하는 데이터 구조 중 하나로서 여러 개의 데이터를 저장할 수 있는 자료형이다.
- List는 배열(Array)과 비슷하게 index를 이용하여 데이터에 접근할 수 있다.
- 배열(Array)은 저장할 수 있는 데이터의 개수가 한 번에 정해지고 바꿀 수 없지만, List는 저장할 수 있는 데이터의 개수를 (동적으로) 변화시킬 수 있어서 데이터의 추가(add), 삭제(remove) 등에 편리하다.
- 따라서 저장할 데이터의 개수가 계속 변하는 상황이라면 배열(Array)보다 List를 사용해야 한다.
- import java.util.List; 로 List 클래스를 불러오는 코딩이 먼저 선행되어야 한다.
[2] ArrayList 란?
- ArrayList는 List interface를 상속받은 클래스 이다
- List 자료형에서 가장 일반적으로 사용하는 유형이 ArrayList이다.
- List의 자료형에는 ArrayList, LinkedList, Vector가 있다.
- 배열과 동일하게 index는 0부터 시작한다.
- import java.util.ArrayList; 로 ArrayList 클래스를 불러오는 코딩이 먼저 선행되어야 한다.
import java.util.List; // import just the List interface
import java.util.ArrayList; // import just the ArrayList class
import java.util.*; // import everything including List and ArrayList
[예제1] List를 선언(Declare)하고 만드는(Creating) 코드에 대해 아래의 내용을 살펴보세요.
import java.util.*;
public class Main {
public static void main(String[] args) {
// [1] Declaring Lists
// nameList that can refer to a list of strings,
// but currently doesn’t refer to any list yet (is set to null).
// List<Type> variable = null;
List<String> stringList1 = null;
List<Integer> integerList1 = null;
System.out.println(stringList1);
System.out.println(integerList1);
// [2] Creating Lists
// List<Type> variable = new ArrayList<Type>();
List<String> nameList2 = new ArrayList<String>(); // 비어있는(empty) list
System.out.println("The size of nameList is: " + nameList2.size()); // size 0
List<String> list2 = null; // not refer to any list
System.out.println("The size of list2 is: " + list2.size()); // size error
List<String> bookList = new ArrayList<String>();
List<Integer> priceList = new ArrayList<Integer>();
}
}
- 배열(Array)의 크기는 .length 로 알 수 있지만, List의 크기는 .size() 메소드로 알 수 있습니다.
- List 선언(Declaring)은 단순히 어떤 List 객체를 참조(가리키다, refer)할 수 있는 변수(variable)를 만든것에 불과합니다. List 선언 자체가 List를 만든(Creating)것은 아닙니다.
- new ArrayList<Type>() 를 사용해야 진짜 List 기능을 하는 List를 만든(Creating)것 입니다.
- <Type>을 넣지 않으면 자동적으로 Object형으로 설정됩니다.
- List의 크기를 얻는 방법은 .size() 메소드
- List가 null로 되어 있을 때 List의 사이즈를 알려고 size()메소드를 사용하면 NullPointerException 에러가 발생합니다.
- List가 null 이라는 의미는 아무런 객체(object)도 참조(refer)하고 있지 않는다는 것이라서, List 객체가 존재하지 않아 List크기값을 알려고 해도 에러가 발생합니다.
- 방식1 : ArrayList<String> pitches = new ArrayList<String>();
- 방식2 : ArrayList<String> pitches = new ArrayList<>(); (뒷 부분의 자료형은 명확하므로 굳이 적지 않아도 된다.)
[유제1] 다음의 조건을 만족하는 ArrayList를 만드세요.
Type | Variable |
String | bookList |
Integer | priceList |
NO | 메소드 | 설명 |
1 | add() | List에 데이터 추가 |
2 | get() | List의 데이터 값 가져와서 확인 |
3 | set() | List의 데이터 값 바꾸기 |
4 | remove() | List의 데이터 값 지우기 |
[예제2] List에 데이터 추가하기
import java.util.*;
public class Main {
public static void main(String[] args) {
List<String> nameList = new ArrayList<String>();
nameList.add("Tom");
System.out.println(nameList);
nameList.add("Grace");
System.out.println(nameList);
nameList.add("Tom");
System.out.println(nameList);
System.out.println(nameList.size());
nameList.add(0, "Jane");
System.out.println(nameList);
nameList.add(1, "Paul");
System.out.println(nameList);
}
}
- List는 중복된 데이터를 가질 수 있습니다.
- add(index, data)를 이용해 data를 List의 특정 위치(index)에 넣으면, 오른쪽 나머지 data들은 뒤로 밀립니다.
[유제2-1] 아래 코드를 실행했을 때 출력되는 결과를 적어보세요.
List<String> list1 = new ArrayList<String>();
list1.add("Anaya");
list1.add("Layla");
list1.add("Sharrie");
list1.add(1, "Sarah");
System.out.println(list1);
[유제2-2] fruitList 라는 variable의 ArrayList를 하나 만드세요. 이 리스트에 문자열 3개 "apple", "banana", "cherry"를 차례대로 넣고 리스트 항목을 출력하세요. 그리고 마지막에 fruitList의 index 2에 "orange"를 넣은다음 다시한번 리스트 항목을 출력하세요.
[예제3] 정수형(Integer) type의 ArrayList 예시 코드입니다.
import java.util.*;
public class Main {
public static void main(String[] args) {
// [1]
List<Integer> list1 = new ArrayList<Integer>();
list1.add(new Integer(1));
System.out.println(list1);
list1.add(new Integer(2));
System.out.println(list1);
list1.add(1, new Integer(3));
System.out.println(list1);
list1.add(1, new Integer(4));
System.out.println(list1);
System.out.println(list1.size());
System.out.println("==========");
// [2]
List<Integer> list2 = new ArrayList<Integer>();
list2.add(1);
System.out.println(list2);
list2.add(2);
System.out.println(list2);
list2.add(1, 3);
System.out.println(list2);
list2.add(1, 4);
System.out.println(list2);
System.out.println(list2.size());
}
}
- Lists can only hold objects, not primitive values.
- This means that int values must be wrapped into Integer objects to be stored in a list.
- You can do this using new Integer(value) as shown above.
- You can also just put an int value in a list and it will be changed into an Integer object automatically. This is called autoboxing.
- When you pull an int value out of a list of Integers that is called unboxing.
[유제3-1] 아래 코드의 실행 결과를 적어보세요.
List<Integer> list1 = new ArrayList<Integer>();
list1.add(new Integer(1));
list1.add(new Integer(2));
list1.add(new Integer(3));
list1.add(2, new Integer(4));
list1.add(new Integer(5));
System.out.println(list1);
[유제3-2] 아래 코드의 실행 결과를 적어보세요.
List<Integer> list1 = new ArrayList<Integer>();
list1.add(5);
list1.add(4);
list1.add(3);
list1.add(1, 2);
System.out.println(list1);
[유제3-3] 아래 코드의 실행 결과를 적어보세요.
List<Integer> list1 = new ArrayList<Integer>();
list1.add(1);
list1.add(3);
list1.add(2);
list1.add(1);
System.out.println(list1);
[예제4] get(), set() 메소드를 List에서 사용해보기
import java.util.*;
public class Main {
public static void main(String[] args) {
List<String> nameList = new ArrayList<String>();
nameList.add("Diego");
nameList.add("Grace");
nameList.add("Deja");
System.out.println(nameList);
System.out.println(nameList.get(0));
System.out.println(nameList.get(1));
System.out.println(nameList.get(2));
nameList.set(1, "John");
System.out.println(nameList);
}
}
- Remember that you can get the value at an array index using value = arrayName[index]
- This is different from how you get the value from a list using obj = listName.get(index)
- You can set the value at an index in an array using arrayName[index] = value
- but with lists you use listName.set(index, object)
[유제4-1] 아래 코드의 실행 결과를 적어보세요.
List<Integer> list1 = new ArrayList<Integer>();
list1.add(new Integer(1));
list1.add(new Integer(2));
list1.add(new Integer(3));
list1.set(2, new Integer(4));
list1.add(2, new Integer(5));
list1.add(new Integer(6));
System.out.println(list1);
[유제4-2] 아래 코드의 실행 결과를 적어보세요.
List<String> list1 = new ArrayList<String>();
list1.add("Anaya");
list1.add("Layla");
list1.add("Sharrie");
list1.set(1, "Destini");
list1.add(1, "Sarah");
System.out.println(list1);
[예제5] remove()메소드로 List의 항목을 삭제해보자.
import java.util.*;
public class Main {
public static void main(String[] args) {
List<Integer> list1 = new ArrayList<Integer>();
list1.add(3);
list1.add(5);
list1.add(7);
System.out.println(list1);
list1.remove(1); // remove(index)
System.out.println(list1);
}
}
- remove 메서드에는 2가지 방식이 있다.
- remove(객체)
- remove(인덱스)
- 첫 번째 방식인 remove(객체)를 사용하면 리스트에서 객체에 해당하는 항목을 삭제한 뒤, 그 결과로 true 또는 false를 리턴한다.
- System.out.println(pitches.remove("129")); // 129를 리스트에서 삭제하고, true를 리턴한다.
- 두 번째 방식인 remove(인덱스)를 사용하면 인덱스에 해당하는 항목을 삭제한 뒤, 그 항목을 리턴한다.
- System.out.println(pitches.remove(0)); // pitches의 첫 번째 항목이 138이므로, 138을 삭제한 뒤 138을 리턴한다.
[유제5-1] 아래 코드의 실행 결과를 적어보세요.
List<Integer> list1 = new ArrayList<Integer>();
list1.add(new Integer(1));
list1.add(new Integer(2));
list1.add(new Integer(3));
list1.remove(1);
System.out.println(list1);
[유제5-2] 아래 코드의 실행 결과를 적어보세요.
List<Integer> list1 = new ArrayList<Integer>();
list1.add(new Integer(1));
list1.add(new Integer(2));
list1.add(new Integer(3));
list1.remove(2);
System.out.println(list1);
[예제6] contains() 메소드를 이용해 List의 항목을 검사해보자.
import java.util.*;
public class Main {
public static void main(String[] args) {
List<Integer> list1 = new ArrayList<Integer>();
list1.add(3);
list1.add(5);
list1.add(7);
boolean test = list1.contains(7);
System.out.println(test);
}
}
- contains 메서드는 리스트 안에 해당 항목이 있는지 판별해 그 결과를 boolean값(true/false)로 리턴한다
[유제6] String형 ArryaList 하나를 만들고, 이 리스트에 "apple, "banana", "cherry"를 추가하세요. 그리고 이 리스트에 "banana"라는 단어가 있는지 contains()메서드로 확인하여 아래와 같이 contains() 리턴값을 출력하세요.
[유제 정답은 아래 "더보기"클릭]
[유제1 정답]
import java.util.*;
public class Main {
public static void main(String[] args) {
List<String> bookList = new ArrayList<String>();
List<Integer> priceList = new ArrayList<Integer>();
}
}
[유제2-1 정답]
["Anaya", "Sarah", "Layla", "Sharrie"]
[유제2-2 정답]
import java.util.*;
public class Main {
public static void main(String[] args) {
List<String> fruitList = new ArrayList<String>();
fruitList.add("apple");
fruitList.add("banana");
fruitList.add("cherry");
System.out.println(fruitList);
fruitList.add(2, "orange");
System.out.println(fruitList);
}
}
[유제3-1 정답]
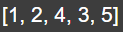
[유제3-2 정답]
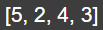
[유제3-3 정답]
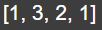
[유제4-1 정답]
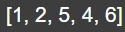
[유제4-2 정답]

[유제5-1 정답]

[유제5-2 정답]

[유제6 정답]
import java.util.*;
public class Main {
public static void main(String[] args) {
List<String> list1 = new ArrayList<String>();
list1.add("apple");
list1.add("banana");
list1.add("cheery");
boolean test = list1.contains("banana");
System.out.println(test);
}
}
'자바(Java) > 자바기초' 카테고리의 다른 글
[자바기초.011] 배열과 리스트 비교(Array vs List) (0) | 2024.03.13 |
---|---|
[자바기초.010] Traversing ArrayList(리스트 반복문) (0) | 2024.03.11 |
[자바기초] 2차원 배열(2D Array) (0) | 2024.03.03 |
[자바기초]1차원 배열(array) (0) | 2024.03.01 |
[자바기초.006]코드업100제_기초출력풀이_1001~1007 (0) | 2022.09.12 |
댓글