[자바AP연습문제]06.Arrays
*06.Arrays에 대한 복습은 아래 "더보기" 클릭
An array is consecutive storage for multiple items of the same type like the top five scores in a game.
You learned how to declare arrays, create them, and access array elements. Array elements are accessed using an index. The first element in an array is at index 0.
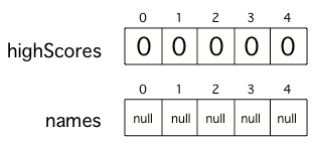
- Array - An array can hold many items (elements) of the same type. You can access an item (element) at an index and set an item (element) at an index.
- Array Declaration - To declare an array specify the type of elements that will be stored in the array, then ([ ]) to show that it is an array of that type, then at least one space, and then a name for the array. Examples: int[ ] highScores; String[ ] names;
- Array Creation - To create an array type the name and an equals sign then use the new keyword, followed by a space, then the type, and then in square brackets the size of the array (the number of elements it can hold). Example: names = new String[5];
- Array Index - You can access and set values in an array using an index. The first element in an array called arr is at index 0 arr[0]. The last element in an array is at the length minus one - arr[arr.length - 1].
- Array Initialization - You can also initialize (set) the values in the array when you create it. In this case you don’t need to specify the size of the array, it will be determined from the number of values that you specify. Example: int[ ] highScores = {99,98,98,88,68};
- Array Length - The length of an array is the number of elements it can hold. Use the public length field to get the length of the array. Example: given int[ ] scores = {1,2,2,1,3,1};, scores.length equals 6.
- Element Reference - A specific element can be referenced by using the name of the array and the element’s index in square brackets. Example: scores[3] will return the 4th element (since index starts at 0, not 1). To reference the last element in an array, use array[array.length - 1]
- For-each Loop - Used to loop through all elements of an array. Each time through the loop the loop variable will be the next element in the array starting with the element at index 0, then index 1, then index 2, etc.
(more detail about "for-each" loop => https://wooduino.tistory.com/220 - Out of Bounds Exception - An error that means that you tried to access an element of the array that doesn’t exist maybe by doing arr[arr.length]. The first valid indices is 0 and the last is the length minus one.
- for - starts both a general for loop and a for-each loop. The syntax for a for each loop is for (type variable : array). Each time through the loop the variable will take on the next value in the array. The first time through the loop it will hold the value at index 0, then the value at index 1, then the value at index 2, etc.
- static - used to create a class method, which is a method that can be called using the class name like Math.abs(-3).
*Common mistakes with arrays
- forgetting to create the array - only declaring it (int[ ] nums;)
- using 1 as the first index not 0
- using array.length as the last valid index in an array, not array.length - 1.
- using array.length() instead of array.length (not penalized on the free response)
- using array.get(0) instead of array[0] (not penalized on the free response)
- going out of bounds when looping through an array (using index <= array.length). You will get an ArrayIndexOutOfBoundsException.
- jumping out an loop too early by using one or more return statements before every value has been processed.
[문제1]
Consider the following method. Given an array initialized to {4, 10, 15}, which of the following represents the contents of the array after a call to mystery(array, 2)?
1 2 3 4 | public void mystery(int[] a, int i) { a[i] = a[i-1] * 2; } | cs |
A. [8, 20, 30]
B. [4, 8, 15]
C. [8, 10, 15]
D. [4, 10, 20]
E. [4, 8, 30]
[문제2]
Consider the following method. Which of the following code segments, appearing in the same class as the mystery method, will result in array2 having the contents {5, 10, 20}?
1 2 3 4 5 | public int[] mystery(int[] a, int i, int value) { a[i + 1] = a[i] + value; return a; } | cs |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | [A] int[] array1 = {5, 10, 15}; int[] array2 = mystery(array1, 0, 10); [B] int[] array1 = {5, 15, 20}; int[] array2 = mystery(array1, 0, 0); [C] int[] array1 = {5, 10, 15}; int[] array2 = mystery(array1, 1, 10); [D] int[] array1 = {5, 15, 20}; int[] array2 = mystery(array1, 2, 0); [E] int[] array1 = {5, 10, 15}; int[] array2 = mystery(array1, 1, 20); | cs |
[문제3]
Which index is the last element in an array called nums at?
A. nums.length
B. nums.length - 1
C. nums.length()
D. nums.length() - 1
E. nums.size() - 1
[문제4]
Which of the following declarations will cause a compile time error?
A. int[] scores = null;
B. int[] scoreArray = {50,90,85};
C. String[] nameArray = new String[10];
D. String[] nameArray = {5, 3, 2};
E. int[] scores = new int[5];
[문제5]
What is returned from arr[3] if arr={6, 3, 1, 2}?
A. 1
B. 2
C. 3
D. 6
E. 4
[문제6]
What is returned from mystery when it is passed {10, 30, 30, 60}?
1 2 3 4 5 6 7 8 9 | public static double mystery(int[] arr) { double output = 0; for (int i = 0; i < arr.length; i++) { output = output + arr[i]; } return output / arr.length; } | cs |
A. 17.5
B. 30.0
C. 130
D. 32
E. 32.5
[문제7]
Given the following values of a and the method doubleLast what will the values of a be after you execute: doubleLast()?
1 2 3 4 5 6 7 8 9 10 | private int[ ] a = {-10, -5, 1, 4, 8, 30}; public void doubleLast() { for (int i = a.length / 2; i < a.length; i++) { a[i] = a[i] * 2; } } | cs |
A. {-20, -10, 2, 8, 16, 60}
B. {-20, -10, 2, 4, 8, 30}
C. {-10, -5, 1, 8, 16, 60}
D. {-10, -5, 1, 4, 8, 30}
[문제8]
What are the values in a after multAll(3) executes?
1 2 3 4 5 6 7 8 9 10 11 | private int[ ] a = {1, 3, -5, -2}; public void multAll(int amt) { int i = 0; while (i < a.length) { a[i] = a[i] * amt; i++; } // end while } // end method | cs |
A. {1, 3, -5, -2}
B. {3, 9, -15, -6}
C. {2, 6, -10, -4}
D. The code will never stop executing due to an infinite loop
[문제9]
What are the values in a after mult(2) executes?
1 2 3 4 5 6 7 8 9 10 | private int[ ] a = {1, 3, -5, -2}; public void mult(int amt) { int i = 0; while (i < a.length) { a[i] = a[i] * amt; } // end while } // end method | cs |
A. {1, 3, -5, -2}
B. {3, 9, -15, -6}
C. {2, 6, -10, -4}
D. The code will never stop executing due to an infinite loop
[문제10]
Which of the following statements is a valid conclusion. Assume that variable b is an array of k integers and that the following is true:
b[0] != b[i] for all i from 1 to k-1
A. The value in b[0] does not occur anywhere else in the array
B. Array b is sorted
C. Array b is not sorted
D. Array b contains no duplicates
E. The value in b[0] is the smallest value in the array
[문제11]
Consider the following code segment. Which of the following statements best describes the condition when it returns true?
1 2 3 4 5 6 | boolean temp = false; for (int i = 0; i < a.length; i++) { temp = (a[i] == val); } return temp; | cs |
A. whenever the first element in a is equal to val
B. Whenever a contains any element which equals val
C. Whenever the last element in a is equal to val
D. Whenever more than 1 element in a is equal to val
E. Whenever exactly 1 element in a is equal to val
[문제12]
Consider the following data field and method findLongest. Method findLongest is intended to find the longest consecutive block of the value target occurring in the array nums; however, findLongest does not work as intended. For example given the code below the call findLongest(10) should return 3, the length of the longest consecutive block of 10s. Which of the following best describes the value actually returned by a call to findLongest?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | private int[] nums = {7, 10, 10, 15, 15, 15, 15, 10, 10, 10, 15, 10, 10}; public int findLongest(int target) { int lenCount = 0; // length of current consecutive numbers int maxLen = 0; // max length of consecutive numbers for (int k = 0; k < nums.length; k++) { if (nums[k] == target) { lenCount++; } else if (lenCount > maxLen) { maxLen = lenCount; } } if (lenCount > maxLen) { maxLen = lenCount; } return maxLen; } | cs |
A. It is the length of the shortest consecutive block of the value target in nums
B. It is the length of the array nums
C. It is the length of the first consecutive block of the value target in nums
D. It is the number of occurrences of the value target in nums
E. It is the length of the last consecutive block of the value target in nums
[문제13]
Consider the following data field and method. Which of the following best describes the contents of myStuff in terms of m and n after the following statement has been executed?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | private int[] myStuff; //precondition: myStuff contains // integers in no particular order public int mystery(int num) { for (int k = myStuff.length - 1; k >= 0; k--) { if (myStuff[k] < num) { return k; } } return -1; } int m = mystery(n) | cs |
A. All values in positions m+1 through myStuff.length-1 are greater than or equal to n.
B. All values in position 0 through m are less than n.
C. All values in position m+1 through myStuff.length-1 are less than n.
D. The smallest value is at position m.
E. The largest value that is smaller than n is at position m.
[문제14]
Consider the following field arr and method checkArray. Which of the following best describes what checkArray returns?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | private int[] arr; // precondition: arr.length != 0 public int checkArray() { int loc = arr.length / 2; for (int k = 0; k < arr.length; k++) { if (arr[k] > arr[loc]) { loc = k; } } return loc; } | cs |
A. Returns the index of the largest value in array arr.
B. Returns the index of the first element in array arr whose value is greater than arr[loc].
C. Returns the index of the last element in array arr whose value is greater than arr[loc].
D. Returns the largest value in array arr.
E. Returns the index of the largest value in the second half of array arr.
[문제15]
Given the following field and method declaration, what is the value in a[1] when m1(a) is run?
1 2 3 4 5 6 7 | int[] a = {7, 3, -1}; public static int m1(int[] a) { a[1]--; return (a[1] * 2); } | cs |
A. 4
B. 2
C. 12
D. 6
E. 3
[문제16]
Consider the following code. What is the maximum amount of times that HELLO could possibly be printed?
1 2 3 4 5 6 7 | for (int i = 1; i < k; i++) { if (arr[i] < someValue) { System.out.print("HELLO") } } | cs |
A. k - 1
B. k + 1
C. k
D. 1
E. 0
[문제17]
Consider the following method changeArray. An array is created that contains {2, 8, 10, 9, 6} and is passed to changeArray. What are the contents of the array after the changeArray method executes?
1 2 3 4 5 | public static void changeArray(int[] data) { for (int k = data.length - 1; k > 0; k--) data[k - 1] = data[k] + data[k - 1]; } | cs |
A. {2, 6, 2, -1, -3}
B. {-23, -21, -13, -3, 6}
C. {10, 18, 19, 15, 6}
D. This method results in an IndexOutOfBounds exception.
E. {35, 33, 25, 15, 6}
[문제18]
Assume that arr1={1, 5, 3, -8, 6} and arr2={-2, -1, -5, 3, -4} what will the contents of arr1 be after copyArray finishes executing?
1 2 3 4 5 6 7 | public static void copyArray(int[] arr1, int[] arr2) { for (int i = arr1.length / 2; i < arr1.length; i++) { arr1[i] = arr2[i]; } } | cs |
A. [-2, -1, -5, 3, -4]
B. [-2, -1, 3, -8, 6]
C. [1, 5, -5, 3, -4]
D. [1, 5, 3, -8, 6]
E. [1, 5, -2, -5, 2]
[문제 정답은 아래 "더보기" 클릭]
[문제1 정답]
D
(array[2] = array[1] * 2 = 10 * 2 = 20.)
[문제2 정답]
C
(a[i+1] = a[2] = a[i] + value = a[1] + 10 = 10 + 10 = 20.)
[문제3 정답]
B
[문제4 정답]
D
(You can not put integers into an array of String objects.)
[문제5 정답]
B
[문제6 정답]
E
(This sums all the values in the array and then returns the sum divided by the number of items in the array. This is the average.)
[문제7 정답]
C
(It loops from the middle to the end doubling each value. Since there are 6 elements it will start at index 3.)
[문제8 정답]
B
(This code multiplies each value in a by the passed amt which is 3 in this case.)
[문제9 정답]
D
[문제10 정답]
A
(The assertion denotes that b[0] occurs only once, regardless of the order or value of the other array values.)
[문제11 정답]
C
(Because each time through the loop temp is reset, it will only be returned as true if the last value in a is equal to val.)
[문제12 정답]
D
(The variable lenCount is incremented each time the current array element is the same value as the target. It is never reset so it counts the number of occurrences of the value target in nums. The method returns maxLen which is set to lenCount after the loop finishes if lenCount is greater than maxLen.)
[문제13 정답]
A
(Mystery steps backwards through the array until the first value less than the passed num (n) is found and then it returns the index where this value is found. Nothing is known about the elements of the array prior to the index at which the condition is met.)
[문제14 정답]
A
(This code sets loc to the middle of the array and then loops through all the array elements. If the value at the current index is greater than the value at loc then it changes loc to the current index. It returns loc, which is the index of the largest value in the array.)
[문제15 정답]
B
(The statement a[1]--; is the same as a[1] = a[1] - 1; so this will change the 3 to 2. The return (a[1] * 2) does not change the value at a[1].)
[문제16 정답]
A
(This loop will start at 1 and continue until k is reached as long as arr[i] < someValue is true. The last time the loop executes, i will equal k-1, if the condition is always true. The number of times a loop executes is equal to the largest value when the loop executes minus the smallest value plus one. In this case that is (k - 1) - 1 + 1 which equals k - 1.)
[문제17 정답]
E
(This method starts at the last valid index of the array and adds the value of the previous element to the element at index k - 1.)
[문제18 정답]
C
(This loop starts at arr2.length / 2 which is 2 and loops to the end of the array copying from arr2 to arr1.)
'자바(Java) > 자바 AP' 카테고리의 다른 글
APCSA(JAVA) Midterm Test(Unit.01~05) (0) | 2024.03.27 |
---|---|
AP Computer Science A Java Quick Reference (0) | 2024.03.25 |
[자바AP연습문제]05.Writing Classes (0) | 2024.03.24 |
[자바AP.04] 반복문(Iteration) (0) | 2024.03.24 |
[자바AP연습문제]03.Boolean Expressions and If Statements (0) | 2024.03.23 |
댓글