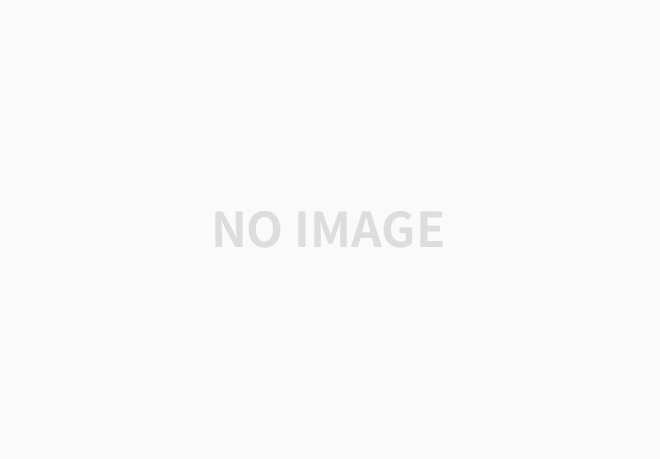
AP Computer Science A Java Quick Reference
(1) String Class
APCSA 시험에서 자주 등장하는 Java method의 이론과 사용 방법에 대한 내용입니다.
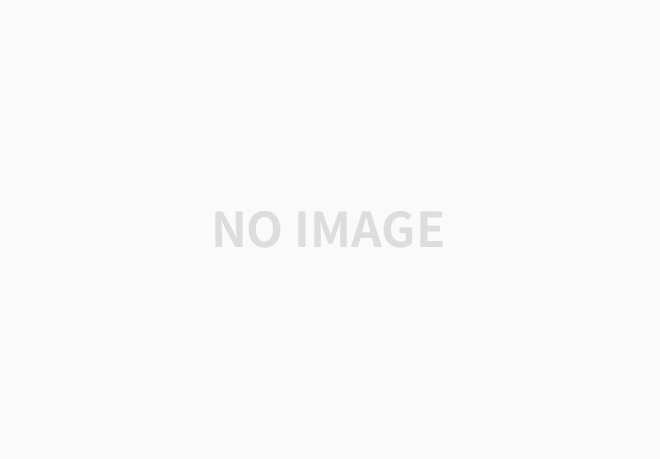
(1) String Class
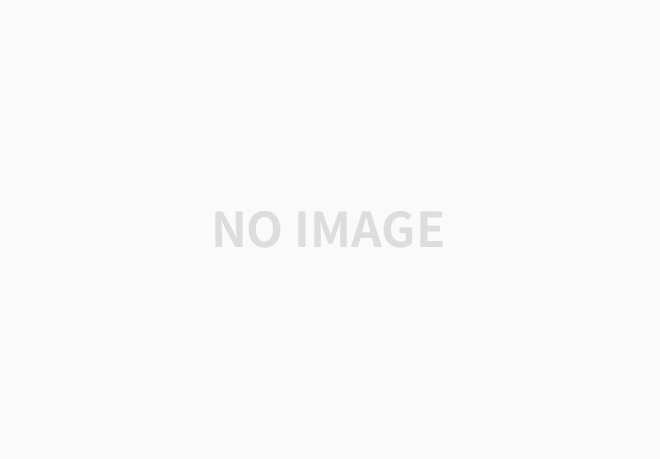
- 자바에는 기본적으로 String 클래스가 존재합니다.
- String은 문자열을 의미하고, 문자열이란 문자(character)가 서로 연결된 형태("Hello")를 말합니다.
- String 클래스로 만든 object들은 String 클래스의 field와 method(위 표 참고)를 모두 사용할 수 있습니다.
[예제1-1]
아래 코드의 실행 결과를 확인해 보세요.
1 2 3 4 5 6 7 8 9 10 11 12 13 | public class Main { public static void main(String[] args) { String greeting1 = null; String greeting2 = "Hello"; String greeting3 = new String("Welcome!"); System.out.println(greeting1); // null System.out.println(greeting2); // Hello System.out.println(greeting3); // Welcome! } } | cs |
- 위 코드에서, greeting1, greeting2와 같이 "string literal"로 String object를 만들수 있다.
- greeting3은 new 키워드를 사용해 String 생성자를 실행하여 String object를 만들었다.
- greeting1 = null처럼 null값이 저장되면 이 object 변수는 어떤 object도 가리키고 있지 않게 되어 println(greeting1)은 null이 출력됩니다.
[예제1-2] 아래 코드의 실행 결과를 확인해 보자.
1 2 3 4 5 6 7 8 9 10 11 12 | public class Main { public static void main(String[] args) { String greeting = "Hello"; Class currentClass = greeting.getClass(); Class parentClass = currentClass.getSuperclass(); System.out.println(currentClass); // class java.lang.String System.out.println(parentClass); // class java.lang.Object } } | cs |
- java.lang은 package 이름이고, 그 package안에 있는 String 클래스를 확인할 수 있다.
- String 클래스의 parent class(superclass)는 Object 클래스이다.
- 자바에 있는 모든 클래스는 Object 클래스를 상속받는다.
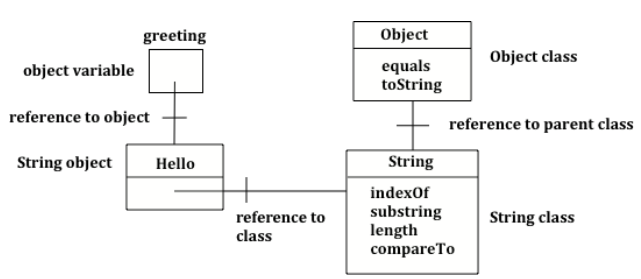
[예제1-3] String 클래스의 문자열 연결(Concatenation)에 대해 아래의 코드로 살펴보세요.
1 2 3 4 5 6 7 8 9 10 11 | public class Main { public static void main(String[] args) { String start = "Happy Birthday"; String name = "Tom"; String result = start + " " + name; // add together strings result += "!"; // add on to the same string System.out.println(result); // Happy Birthday Tom! } } | cs |
- String 문자열은 +, += 를 이용하여 문자열끼리 서로 합칠 수 있고, 이런 것을 "appending" or "concatenating"이라고 부릅니다.
- 다른 종류의 값들(정수형, 실수형 등)을 String과 함께 + or +=로 합치면, 그 다른 종류의 값들이 String으로 자동변환되어 문자열로 합쳐집니다.
[유제1-1]
아래 코드를 실행했을 때, s1의 값은?
1 2 3 | String s1 = "xy"; String s2 = s1; s1 = s1 + s2 + "z"; | cs |
A. xyz
B. xyxyz
C. xy xy z
D. xy z
E. z
[유제1-2] 아래 코드를 실행해보고, () 괄호의 역할을 말해보자.
1 2 3 4 5 6 7 8 9 | public class Main { public static void main(String[] args) { String m1 = "12" + 4 + 3; String m2 = "12" + (4 + 3); System.out.println(m1); // 1243 System.out.println(m2); // 127 } } | cs |
[예제1-4]
큰 따옴표(", double quote)나 \ (backslash)는 Java에서 명령어이기 때문에 이것을 화면에 출력하려면 문자 앞에 \ 를 하나 더 붙여주어야 합니다. 아래의 예제 코드를 실행해 보세요.
1 2 3 4 5 6 7 8 9 | public class Main { public static void main(String[] args) { String message = "Here is a backslash quote \" " + " and a backslashed backslash (\\) " + "Backslash n \n prints out a new line."; System.out.println(message); } } | cs |
[출력결과]

(2) String Methods
[예제2] 아래 코드를 실행해서 String methods중 length(), substring(), indexOf() 3가지의 실행과정에 대해 알아보자.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | public class Main { public static void main(String[] args) { String message1 = "This is a test"; String message2 = "Hello Class"; System.out.println(message1.length()); // 14 System.out.println(message2.length()); // 11 System.out.println(message1.substring(0, 3)); // Thi System.out.println(message1.substring(2, 3)); // i System.out.println(message1.substring(5)); // is a test System.out.println(message1.indexOf("is")); // 2 // This will match the is in "This"! System.out.println(message1.indexOf("Hello")); // -1 System.out.println(message2.indexOf("Hello")); // 0 // lowercase and uppercase are not on the AP exam, but still useful System.out.println(message2.toLowerCase()); // hello class System.out.println(message2.toUpperCase()); // HELLO CLASS } } | cs |
- String Class의 메소드중 length()는 string(문자열)의 문자개수를 리턴해 주는 메소드 입니다.
- substring(start)는 start index에서 시작하여 마지막 index까지 글자를 빼내어 리턴하고, substring(from,to)는 from index에서 시작하여 to index 직전(-1)까지의 글자를 빼내어 리턴해준다.
- string.indexOf(substring) 메소드는 string 문자열에서 내가 원하는 substring을 찾아서 그 index 위치값을 리턴해주는데, 만약 찾는 문자가 존재하지 않으면 -1을 리턴해준다.
[유제2-1]
아래 코드를 실행한 뒤 pos의 값은 얼마인가요?
1 2 | String s1 = "abccba"; int pos = s1.indexOf("b"); | cs |
A. 2
B. 1
C. 4
D. -1
[유제2-2]
아래 코드를 실행한 뒤 len 값은 얼마인가요?
1 2 | String s1 = "baby"; int len = s1.length(); | cs |
A. 2
B. 3
C. 4
D. -1
[유제2-3]
아래 코드를 실행 한 뒤, s2의 값은 얼마인가요?
1 2 | String s1 = "baby"; String s2 = s1.substring(0,3); | cs |
A. baby
B. b
C. ba
D. bab
[유제2-4]
아래 코드를 실행 한 뒤, s2의 값은 얼마인가요?
1 2 | String s1 = "baby"; String s2 = s1.substring(2); | cs |
A. by
B. aby
C. a
D. b
E. ba
(3) CompareTo() and Equals() Methods
- Primitive types(int, double, boolean,...)의 값을 비교할 때는 ==, <, > 같은 것을 사용한다.
- 하지만 Reference type인 String 문자열을 비교할 때는 compareTo(), equals() 같은 메소드를 사용해야 한다.
- s1.compareTo(s2) 메소드는 2개의 문자열(s1, s2)의 글자 하나씩을 모두 비교하여, 정확히 동일한 문자라면 0을 리턴한다. 하지만 문자열 s1의 글자 하나가 s2의 글자보다 알파벳 순서상 앞서면 음수(negative number)를 리턴하고, 뒷서면 양수(positive number)를 리턴한다. (예를 들어, A는 H보다 7칸 떨어져 있다. ABCDEFGH)
- s1.equals(s2) 메소드는 2개의 문자열(s1, s2)를 글자 하나씩 모두 비교하여 같으면 true를, 다르면 false를 리턴한다.
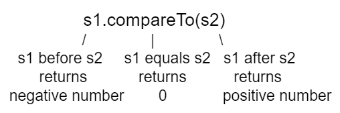
[예제3-1]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | public class Main { public static void main(String[] args) { String str = "abcd"; // 1) 비교대상에 문자열이 포함되어 있을 경우 System.out.println( str.compareTo("abcd") ); // 0 System.out.println( str.compareTo("ab") ); // 2 System.out.println( str.compareTo("a") ); // 3 System.out.println( str.compareTo("c") ); // -2 System.out.println( "".compareTo(str) ); // -4 // 2) 비교대상과 전혀 다른 문자일 경우 System.out.println( str.compareTo("zefd") ); // -25 System.out.println( str.compareTo("zEFd") ); // -25 System.out.println( str.compareTo("ABCD") ); // 32 } } | cs |
- compareTo() 함수에는 "문자열의 비교" 와 "숫자의 비교" 두 방식이 존재한다.
- 숫자의 비교 같은 경우는 단순히 크다(1), 같다(0), 작다(-1) 의 관한 결과값을 리턴해준다.
- 문자열의 비교 같은 경우는 같다(0), 그 외는 문자의 ASCII값을 비교해 양수/음수값을 리턴해 준다.
compareTo()
- int compareTo(NumberSubClass referenceName)
- int compareTo(String anotherString)
- compareTo() 함수는 두개의 값을 비교하여 int 값으로 반환해주는 함수이다.
<compareTo() 숫자형 비교>
1 2 3 4 5 6 7 8 9 | Integer x = 3; Integer y = 4; Double z = 1.0; System.out.println( x.compareTo(y) ); // -1 System.out.println( x.compareTo(3) ); // 0 System.out.println( x.compareTo(2) ); // 1 System.out.println( z.compareTo(2.7) ); // -1 | cs |
위에 예제를 보면 알겠지만 숫자형 비교는 Byte, Double, Integer, Float, Long 또는 Short 등을 비교할 수 있으며, 반환되는 값의 경우 아래의 규칙을 따른다.
기준 값과 비교대상이 동일한 값일 경우 0
기준 값이 비교대상 보다 작은 경우 -1
기준 값이 비교대상 보다 큰 경우 1
예제를 보면 타입을 Integer로 선언해 주었는데, 만약 int타입을 가지고 비교하고자 한다면 다음과 같이 사용하면 된다.
1 2 3 4 5 6 7 | public class Main { public static void main(String[] args) { int x = 4; int y = 5; System.out.println(Integer.compare(x,y)); // -1 } } | cs |
<compareTo() 문자열 비교>
1) 비교대상에 문자열이 포함되어있을 경우
str.compareTo("ab") 의 결과값이 2인 이유는 "abcd" 에 "ab" 가 포함되어있으면 즉,
기준값에 비교대상이 포함되어있을 경우 서로의 문자열 길이의 차이값을 리턴해주기 때문이다.
같은 이유로 아래와 같은 값이 출력되는 것이다.
"abcd"(4) - "ab"(2) = 2,
"abcd"(4) - "a"(1) = 3,
"abcd"(4) - ""(0) = 4
그럼 여기서 하나 의문인게,
왜 str.compareTo("c") 의 결과값이 왜 -2가 나올까? 분명 중간에 포함되어있는데 같은 이유로 길이를 비교해줘야 하는거 아닌가? 아쉽게도 comparTo는 같은 위치의 문자만 비교하기 때문에, 첫번째 문자부터 순서대로 비교해서 다를 경우 바로 아스키값을 기준으로 비교처리를 한다. 비교가 불가능한 지점의 각 문자열의 아스키값을 기준으로 비교를 해준다. "abcd" 와 "c" 를 비교해줄 경우 첫번째 위치에서 비교가 실패했기 때문에,
"a" 와 "c"의 아스키코드 값의 차이값을 리턴해준다. 이둘의 아스키코드의 차이값은 a = 97 / c = 99 이기 때문에 순서에 따라 -2값이 리턴되는것이다.
"abhg".compareTo("h"); // -7
"abcd".compareTo("abfd"); // -3
위의 첫번째 코드는 a = 97 / h = 104 이기 때문에 차이값은 -7이 되는것이다.
두번째 코드는 비교가 불가능한 시점을 찾아줘야 하는데, 여기서 비교가 불가능한 시점이 어디일까? ab는 서로 동일하기에 c와 f의 비교에서 비교가 불가능하다. 그렇기 때문에 c = 99 / f =102 이기 때문에 차이값은 -3이 되는것이다.
2) 비교대상과 전혀 다른 문자열인 경우
비교대상과 전혀 다른 문자일 경우 역시 크게 다를거 없다. 이 역시도 위에서 설명한것과 마찬가지로 비교가 불가능한 지점의 문자열 아스키값을 기준으로 비교한다. str.compareTo("zefd") 이것의 값은 -25가 나오는데 그 이유는 모두 짐작한거와 같이 a = 97 / z = 122 이기 때문에 차이값인 -25가 반환되며 str.compareTo("ABCD") 이것과 같은 경우는 compareTo의 경우 대소문자를 구분하기 때문에 a = 97 / A = 65 이므로 차이값인 32가 반환되는 것이다. (여기서 대소문자를 무시하고 비교해주는 함수 compareToIgnorecase() 가 존재한다.)
[예제3-2]
1 2 3 4 5 6 7 8 | String message = "Hello!"; System.out.println(message.compareTo("Hello!")); // 0 System.out.println(message.compareTo("And")); // 7 System.out.println(message.compareTo("Zoo")); // -18 System.out.println(message.equals("Hello!")); // true System.out.println(message.equals("hello!")); // false | cs |
[유제3-1]
아래의 코드를 실행한 뒤 s2의 값은 얼마인가요?
1 2 3 | String s1 = new String("hi there"); int pos = s1.indexOf("e"); String s2 = s1.substring(0,pos); | cs |
A. hi th
B. hi the
C. hi ther
D. hi there
[유제3-2]
아래의 코드를 실행한 뒤 s1의 값은 얼마인가요?
1 2 3 | String s1 = "Hi"; String s2 = s1.substring(0,1); String s3 = s2.toLowerCase(); | cs |
A. Hi
B. hi
C. H
D. h
[유제3-3]
아래의 코드를 실행한 뒤 s3의 값은 얼마인가요?
1 2 3 | String s1 = "Hi"; String s2 = s1.substring(0,1); String s3 = s2.toLowerCase(); | cs |
A. Hi
B. hi
C. H
D. h
[유제3-4]
아래 코드를 실행 한 뒤, answer의 값은 얼마인가요?
1 2 3 | String s1 = "Hi"; String s2 = "Bye"; int answer = s1.compareTo(s2); | cs |
A. positive (> 0)
B. 0
C. negative (< 0)
*단원 내용 요약* (아래 더보기 클릭)
index - A number that represents the position of a character in a string. The first character in a string is at index 0.
length - The number of characters in a string.
substring - A new string that contains a copy of part of the original string.
A String object has index values from 0 to length –1. Attempting to access indices outside this range will result in an IndexOutOfBoundsException.
String objects are immutable, meaning that String methods do not change the String object. Any method that seems to change a string actually creates a new string.
The following String methods and constructors, including what they do and when they are used, are part of the AP CSA Java Quick Reference Sheet that you can use during the exam:
String(String str) : Constructs a new String object that represents the same sequence of characters as str.
int length() : returns the number of characters in a String object.
String substring(int from, int to) : returns the substring beginning at index from and ending at index (to -1).
String substring(int from) : returns substring(from, length()).
int indexOf(String str) : searches for str in the current string and returns the index of the first occurrence of str; returns -1 if not found.
boolean equals(String other) : returns true if this (the calling object) is equal to other; returns false otherwise.
int compareTo(String other) : returns a value < 0 if this is less than other; returns zero if this is equal to other; returns a value > 0 if this is greater than other.
str.substring(index, index + 1) returns a single character at index in string str.
[유제 정답은 아래 "더보기" 클릭]
[유제1-1 정답]
B
[유제1-2 정답]
()괄호는 정수의 +연산을 String concatenating보다 먼저 실행되게 해준다.
[유제2-1 정답]
B
(The method indexOf returns the first position of the passed str in the current string starting from the left (from 0))
[유제2-2 정답]
C
(Length returns the number of characters in the string.)
[유제2-3 정답]
D
(Substring returns all the characters from the starting index to the last index -1.)
[유제2-4 정답]
A
(The method substring(index) will return all characters starting the index to the end of the string.)
[유제3-1 정답]
A
(The substring method returns the string starting at the first index and not including the last index. The method indexOf returns the index of the first place the string occurs.)
[유제3-2 정답]
A
(Strings are immutable, meaning they don't change. Any method that changes a string returns a new string. So s1 never changes.)
[유제3-3 정답]
D
(s2 is set to just "H" and s3 is set to changing all characters in s2 to lower case.)
[유제3-4 정답]
A
(H is after B in the alphabet so s1 is greater than s2.)
'자바(Java) > 자바 AP' 카테고리의 다른 글
AP Computer Science A Java Quick Reference (2) Integer & Double (0) | 2024.03.28 |
---|---|
APCSA(JAVA) Midterm Test(Unit.01~05) (0) | 2024.03.27 |
[자바AP연습문제]06.Arrays (0) | 2024.03.24 |
[자바AP연습문제]05.Writing Classes (0) | 2024.03.24 |
[자바AP.04] 반복문(Iteration) (0) | 2024.03.24 |
댓글