AP Computer Science A [FRQ] Practice (1)
FRQ Question 1 notice
FRQ Question 1 on Control Structures will probably involve:
-a for-loop that probably uses the method’s parameter variables,
-an if statement, probably inside the loop,
-calls to other class methods given to you,
-a numerical or string value that is calculated by the loop and returned at the end of the method.
-if the question has 2 parts, 1 part will probably require a loop and the other just an expression.
On question 1, you will get points for:
-constructing the loop correctly,
-calling the correct methods,
-calculating the correct value,
-and returning the value.
Try to have some code for each of these steps. Do not use arrays or other more complex code. This question does not involve more complex topics such as arrays. You may need to use Math or string methods.
*Java Quick Reference(아래 "더보기")
*2019 FRQ 출시문제
위 문제의 Question 1 부분을 풀어보세요.
Question 1 부분만 캡쳐한 것을 보려면 아래 "더보기" 클릭
Question 1의 해설과 답은 아래 "더보기" 클릭
Solution of Question (1)
(a)
먼저 코딩해야 하는 메소드에서 리턴해야 할 변수, 그 변수의 type을 먼저 코딩을 하자.(이것만으로도 부분점수가 가능하기 때문)
그래서 numberOfLeapYears 메소드의 리턴 type은 int이고, 리턴해야 할 변수는 윤년의 개수(number of leap years)이기 때문에 아래와 같이 먼저 코딩해 줍니다.
1 2 3 4 5 6 7 8 9 10 | /** Returns the number of leap years between year1 and year2, inclusive. * Precondition: 0 <= year1 <= year2 */ public static int numberOfLeapYears(int year1, int year2) { int numLeapYears = 0; // Your loop will go in here return numLeapYears; } | cs |
(quiz)
Which loop should you use to count the number of leap years between year1 and year2?
=> for loop
(in this case, for loop is easier than while loop, you should use for loop when you know how many times the loop should excute.)
(quiz)
What is the starting and ending values for the loop to count the leap years between year 1 and year 2?
=> Loop from year1 to year2
그래서 for loop를 사용하고, year1 ~ year2 까지의 범위라는 것을 생각해 냈다면 아래와 같이 코딩을 생각해 볼 수 있다.
1 2 3 4 | for(int i = year1 ; i <= year2 ; i++) { } | cs |
그리고 윤년(leap year)인지 판단하는 메소드로, 이미 주어진 isLeapYear()를 if문과 함께 사용할 수 있어야 한다.
1 2 | if (isLeapYear(i)) ... | cs |
(모범답안)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | /** Returns the number of leap years between year1 and year2, inclusive. * Precondition: 0 <= year1 <= year2 */ public static int numberOfLeapYears(int year1, int year2) { /* to be implemented in part (a) */ int numberOfLeapYears = 0; for(int i = year1; i <= year2; i++) { if(isLeapYear(i)) { numberOfLeapYears++; } } return numberOfLeapYears; } | cs |
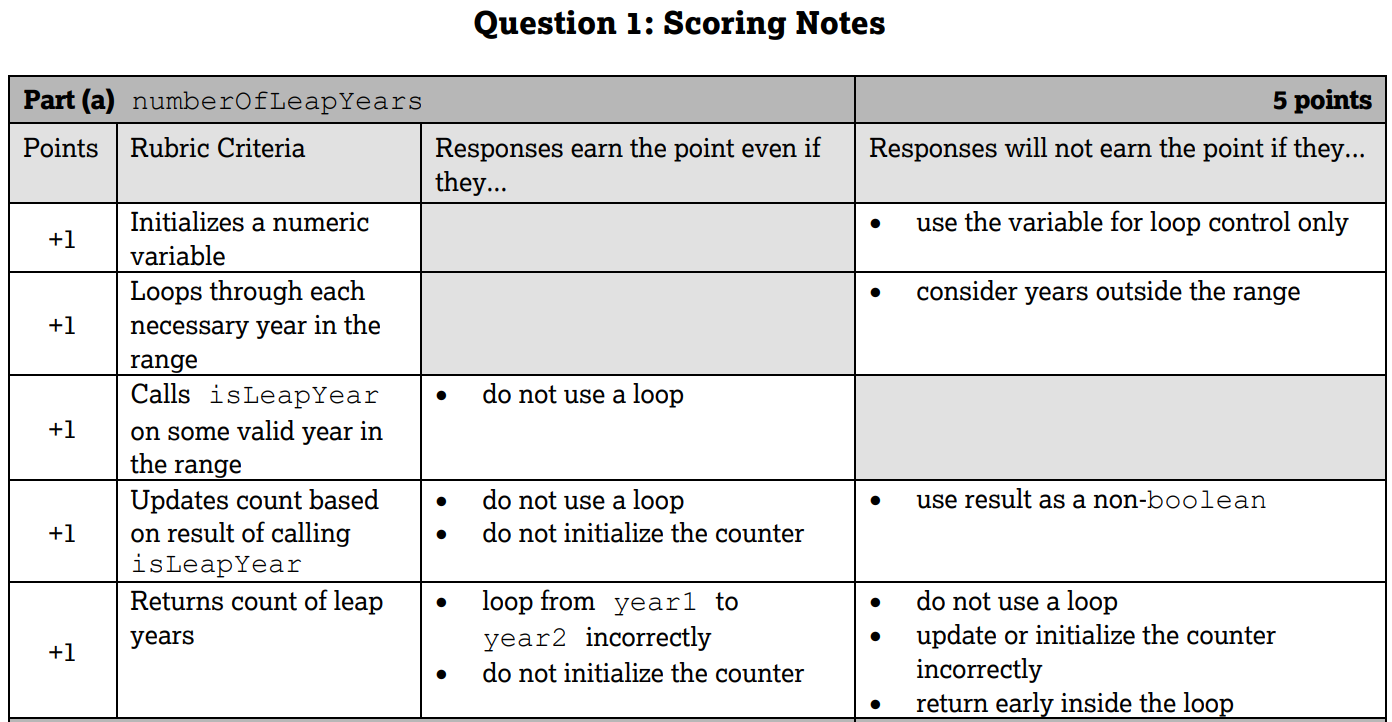
(b)
(quiz)
If firstDayOfYear(2019) returns 2 for a Tuesday for 1/1/2019, what day of the week is Jan. 4th 2019?
=> Friday (5)
(Since 1/1/19 is a Tuesday, Jan. 4th 2019 is 3 days later on a Friday.)
(quiz)
Which of the following expressions return the right value for the day of the week (5) for Jan. 4th 2019 given that firstDayOfYear(2019) returns 2 and dayOfYear(1,4,2019) returns 4?
=> firstDayOfYear(2019) + dayOfYear(1,4,2019) - 1
(You must start at the firstDayOfYear and add on the days following up until that date - 1 since you start counting at 1.)
(quiz)
If firstDayOfYear(2019) returns 2 for a Tuesday for 1/1/2019, what day of the week from (0-6 where 0 is Sunday) is Jan. 8th 2019?
=> Since 1/1/19 is a Tuesday, Jan. 8th 2019 is 7 days later so would fall on the same day of the week.
(모범답안)
1 2 3 4 5 6 7 8 | public static int dayOfWeek(int month, int day, int year) { /* to be implemented in part (b) */ int firstDay = firstDayOfYear(year); int nDay = dayOfYear(month, day, year); int dayOfWeek = (firstDay + nDay - 1) % 7; return dayOfWeek; } | cs |
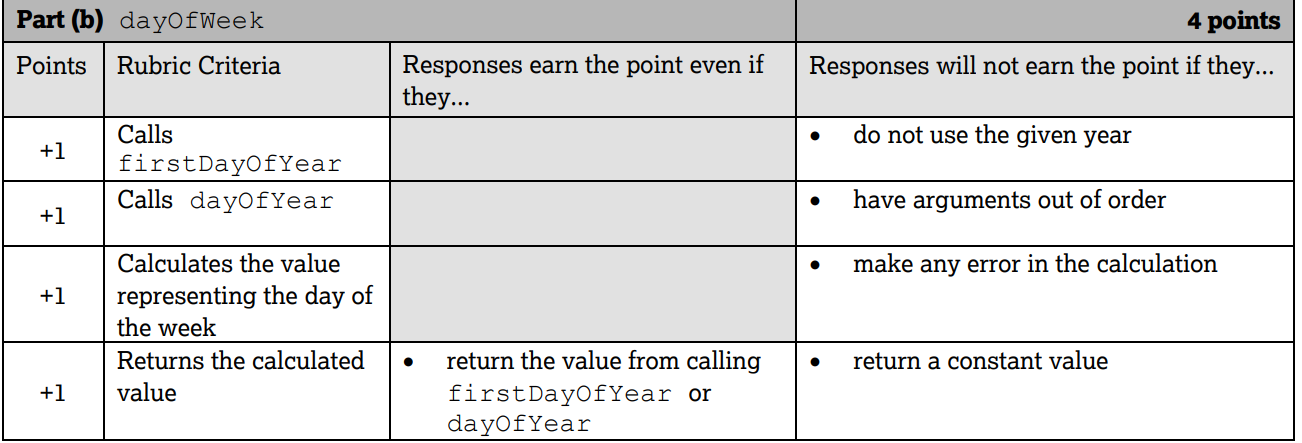
'자바(Java) > 자바 AP' 카테고리의 다른 글
AP Computer Science A [FRQ] Practice (3) (0) | 2024.04.04 |
---|---|
AP Computer Science A [FRQ] Practice (2) (0) | 2024.04.04 |
APCSA Mock Test (0) | 2024.03.29 |
[자바AP연습문제] 10.Recursion (0) | 2024.03.29 |
[자바AP연습문제] 09.Inheritance (0) | 2024.03.29 |
댓글