반응형
AP Computer Science A [FRQ] Practice (3)
FRQ 3번 문제에는 1-D Array나 ArrayList를 활용한 문제가 나옵니다.
아래는 시험과 관련된 주요 알고리즘 입니다.
Here are some common algorithms that you should be familiar with for the AP CSA exam:
- Determine the minimum or maximum value in an array
- Compute a sum, average, or mode of array elements
- Search for a particular element in the array
- Determine if at least one element has a particular property
- Determine if all elements have a particular property
- Access all consecutive pairs of elements
- Determine the presence or absence of duplicate elements
- Determine the number of elements meeting specific criteria
- Shift or rotate elements left or right
- Reverse the order of the elements
Here are two common array traversal loops that can be used for these algorithms:
1 2 3 4 5 6 7 8 9 10 11 | for (int value : array) { if (value ....) ... } for(int i=0; i < array.length; i++) { if (array[i] ....) ... } | cs |
*FRQ 2012 3번 문제
[정답은 아래 "더보기" 클릭]
더보기
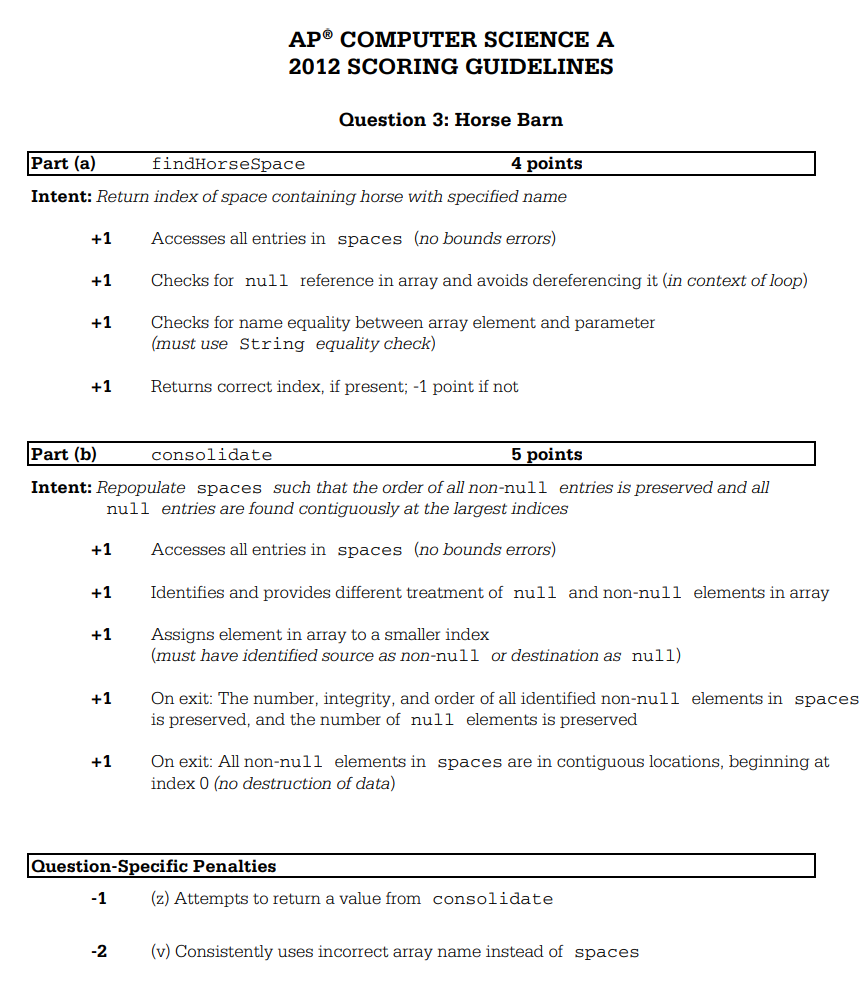
*FRQ 2012 3번 문제 모범답안
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 | // Question 3: Horse Barn // Part (a): public int findHorseSpace(String name) { for (int i = 0; i < this.spaces.length; i++) { if (this.spaces[i]!=null && name.equals(this.spaces[i].getName())) { return i; } } return -1; } //Part (b): public void consolidate() { for (int i = 0; i < this.spaces.length-1; i++) { if (this.spaces[i] == null) { for (int j = i+1; j < this.spaces.length; j++) { if (this.spaces[j] != null) { this.spaces[i] = this.spaces[j]; this.spaces[j] = null; j = this.spaces.length; } } } } } //Part (b): Alternative solution (auxiliary with array) public void consolidate() { Horse[] newSpaces = new Horse[this.spaces.length]; int nextSpot = 0; for (Horse nextHorse : this.spaces) { if (nextHorse != null) { newSpaces[nextSpot] = nextHorse; nextSpot++; } } this.spaces = newSpaces; } //Part (b): Alternative solution (auxiliary with ArrayList) public void consolidate() { List<Horse> horseList = new ArrayList<Horse>(); for (Horse h : this.spaces) { if (h != null) horseList.add(h); } for (int i = 0; i < this.spaces.length; i++) { this.spaces[i] = null; } for (int i = 0; i < horseList.size(); i++) { this.spaces[i] = horseList.get(i); } } | cs |
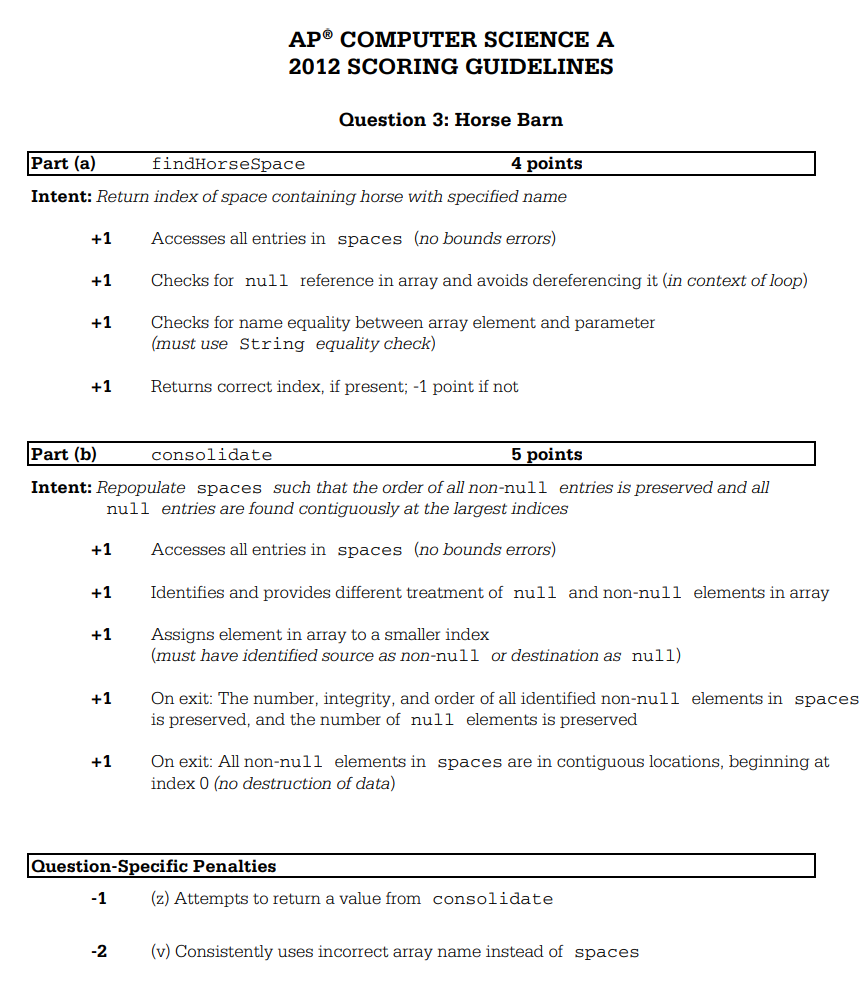
*FRQ 2007 1번 문제(b번만 배열관련 문제)
[정답은 아래 "더보기" 클릭]
더보기
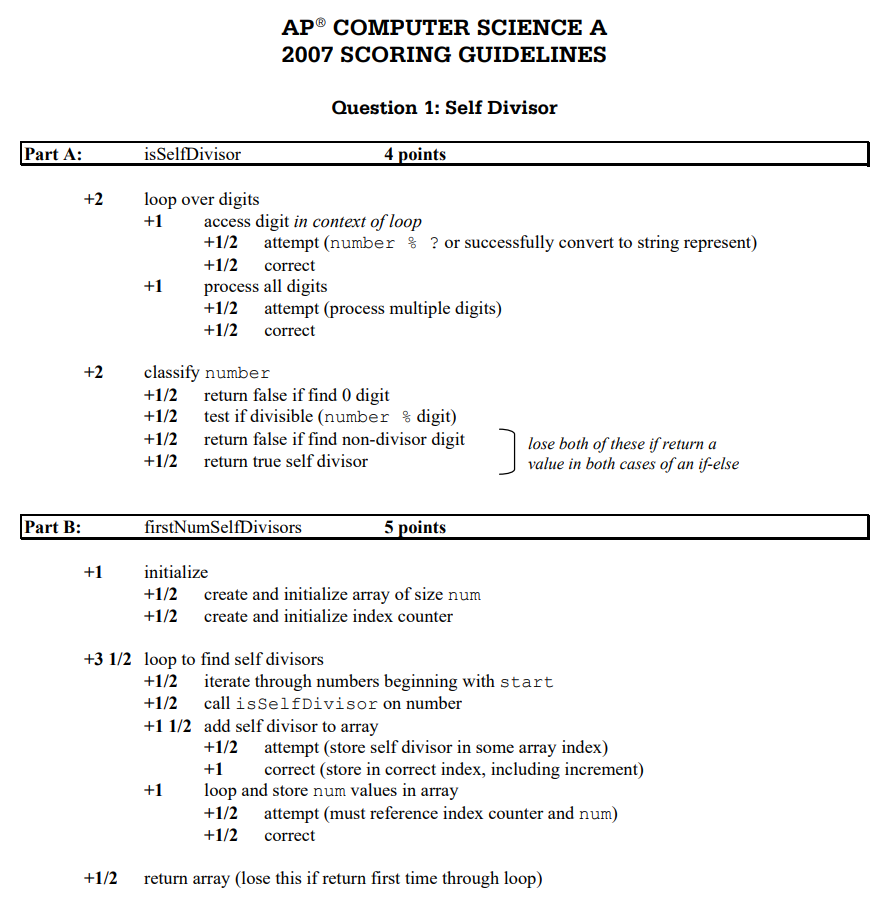
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | // part (a) public static boolean isSelfDivisor(int number) { int n = number; while(n > 0) { int digit = n % 10; if(digit == 0 || number % digit != 0) { return false; } n /= 10; } return true; } // part (b) public static int[] firstNumSelfDivisors(int start, int num) { int[] selfs = new int[num]; int numStored = 0; int nextNumber = start; while(numStored < num) { if(isSelfDivisor(nextNumber)) { selfs[numStored] = nextNumber; numStored++; } nextNumber++; } return selfs; } | cs |
(다르게)
(a)
public class SelfDivisor {
public static boolean isSelfDivisor(int number) {
int originalNumber = number;
while(number > 0) {
int digit = number % 10;
if(digit == 0) return false;
else {
if((originalNumber % digit) == 0) {
number /= 10;
}
else return false;
}
}
return true;
}
}
(b)
public static int[] firstNumSelfDivisors(int start, int num) {
int number = start;
int[] result = new int[num];
int idx = 0;
while(idx < num) {
if(isSelfDivisor(number)) {
result[idx] = number;
idx++;
}
number++;
}
return result;
}
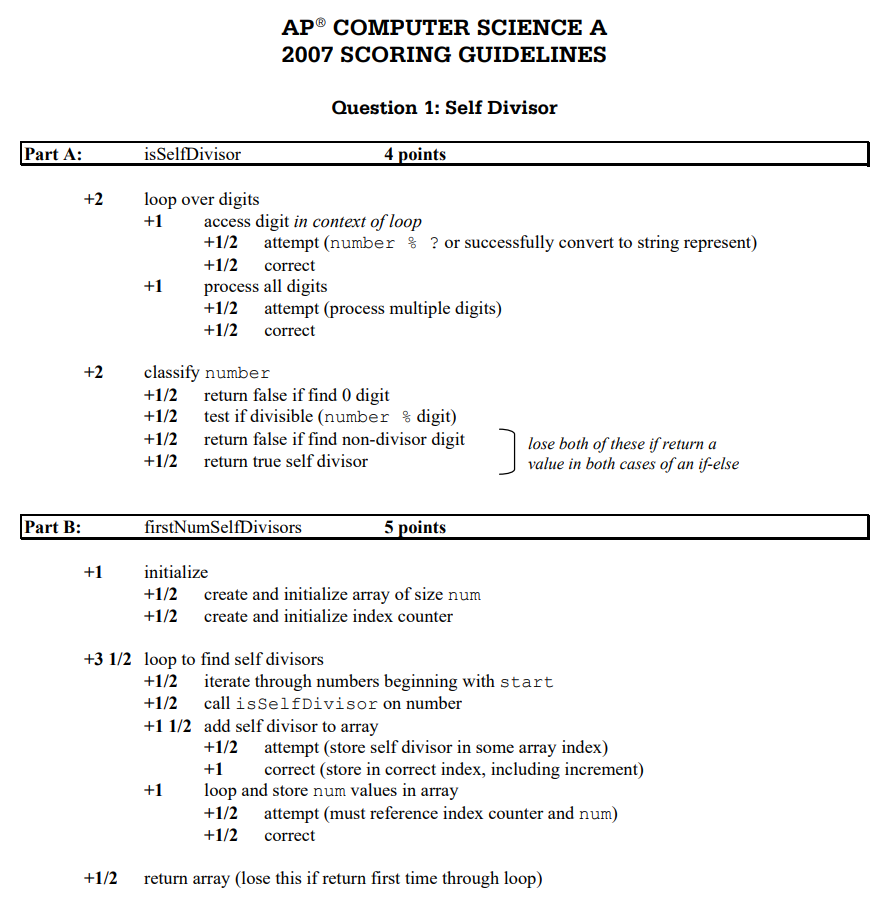
*FRQ 2011 1번 문제
[정답은 아래 "더보기" 클릭]
더보기
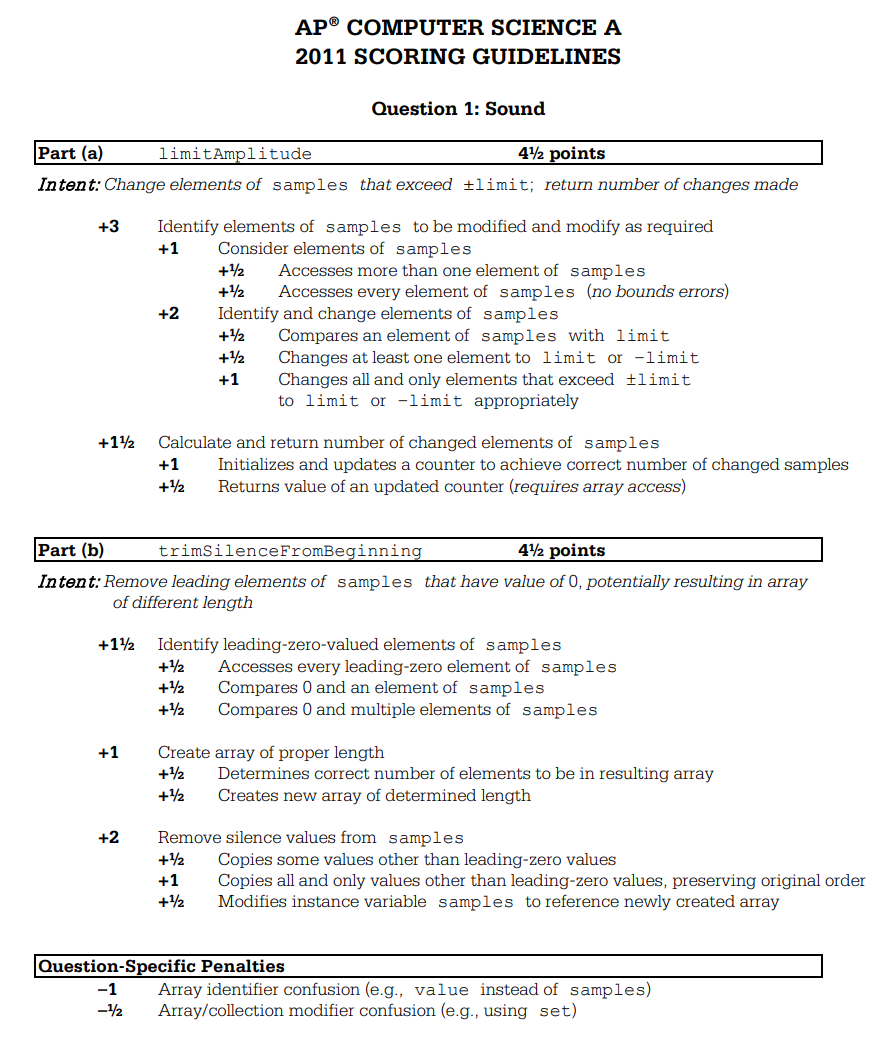
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | //Part (a): public int limitAmplitude(int limit) { int numChanged = 0; for (int i = 0; i < this.samples.length; i++) { if (this.samples[i] < -limit) { this.samples[i] = -limit; numChanged++; } if (this.samples[i] > limit) { this.samples[i] = limit; numChanged++; } } return numChanged; } //Part (b): public void trimSilenceFromBeginning() { int i = 0; while (this.samples[i] == 0) { i++; } int[] newSamples = new int[this.samples.length - i]; for (int j = 0; j < newSamples.length; j++) { newSamples[j] = this.samples[j+i]; } this.samples = newSamples; } | cs |
(다르게)
public class Sound {
private int[] samples;
// (a)
public int limitAmplitude(int limit) {
int numChanged = 0;
for(int i = 0; i < this.samples[i]; i++) {
if(samples[i] > limit) {
samples[i] = limit;
numChanged++;
}
else if(samples[i] < -limit) {
samples[i] = -limit;
numChanged++;
}
}
return numChanged;
}
//(b)
public void trimSilenceFromBeginning() {
int num = this.samples.length;
int[] temp = new int[num];
int idx = 0;
for(int i = 0; i < num; i++) {
if(sample[i] == 0) continue;
else {
temp[idx] = this.sample[i];
idx++;
}
}
this.sample = temp;
}
}
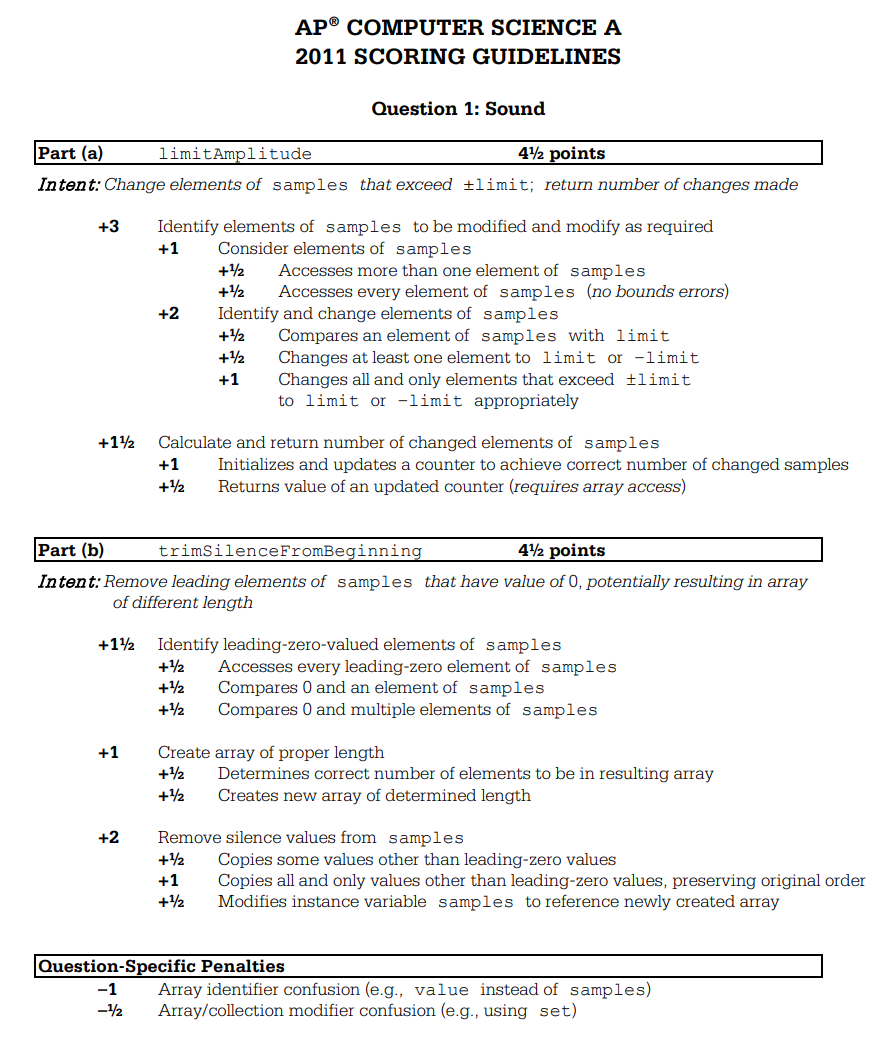
*FRQ 2009 1번 문제
[정답은 아래 "더보기" 클릭]
더보기
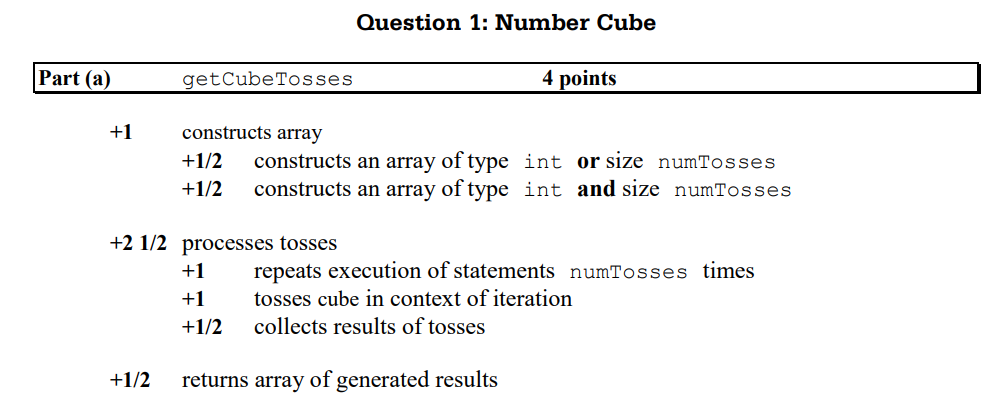
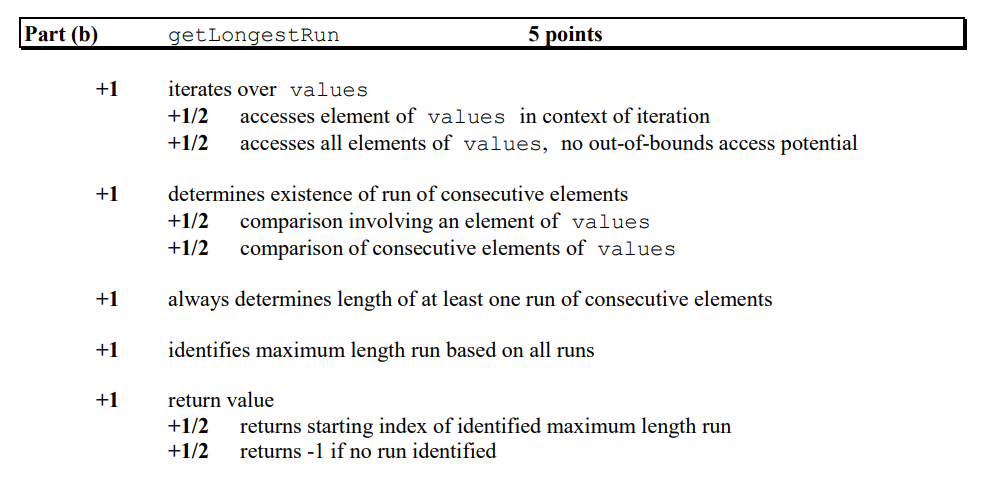
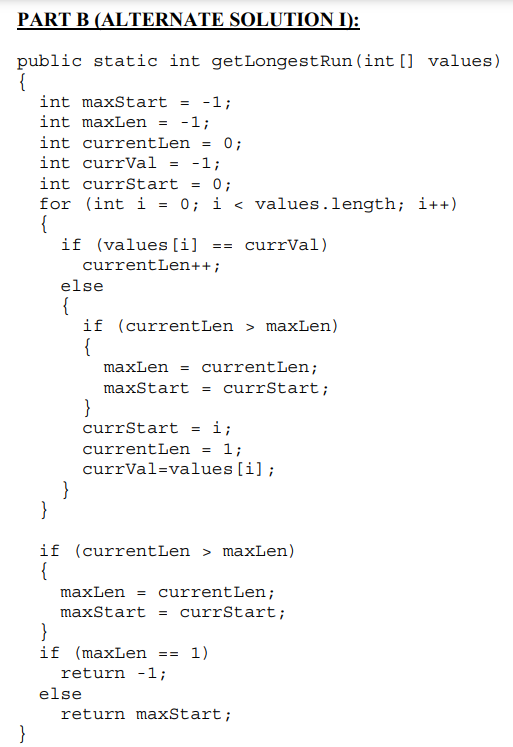
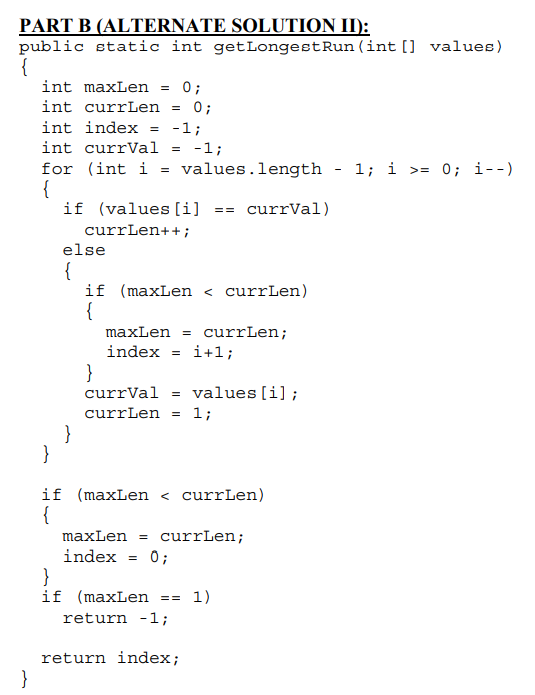
*FRQ 2009 1번 문제 정답
(a)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | PART A: /** Returns an array of the values obtained by tossing * a number cube numTosses times. * @param cube a NumberCube * @param numTosses the number of tosses to be recorded * Precondition: numTosses > 0 * @return an array of numTosses values */ public static int[] getCubeTosses(NumberCube cube, int numTosses) { int[] cubeTosses = new int[numTosses]; for (int i = 0; i < numTosses; i++) { cubeTosses[i] = cube.toss(); } return cubeTosses; } | cs |
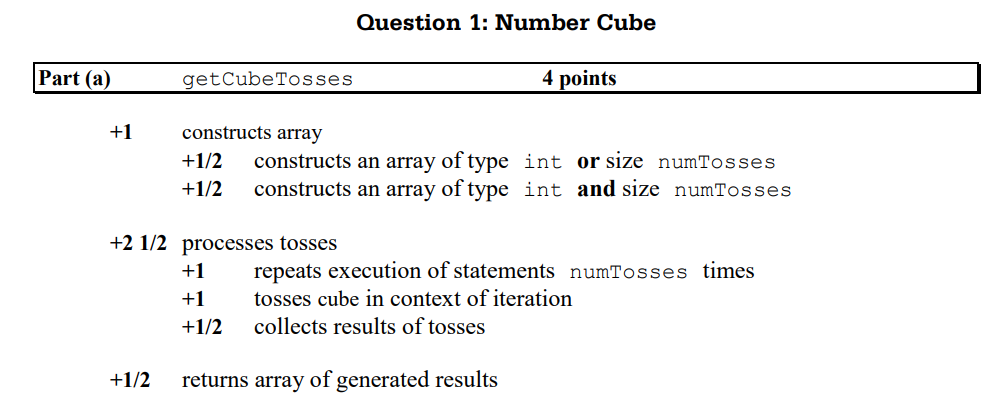
(b)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | PART B: /** Returns the starting index of a longest run of two or more * consecutive repeated values in the array values. * @param values an array of integer values representing a series * of number cube tosses * Precondition: values.length > 0 * @return the starting index of a run of maximum size; * -1 if there is no run */ public static int getLongestRun(int[] values) { int currentLen = 0; int maxLen = 0; int maxStart = -1; for (int i = 0; i < values.length-1; i++) { if (values[i] == values[i+1]) { currentLen++; if (currentLen > maxLen) { maxLen = currentLen; maxStart = i - currentLen + 1; } } else { currentLen = 0; } } return maxStart; } | cs |
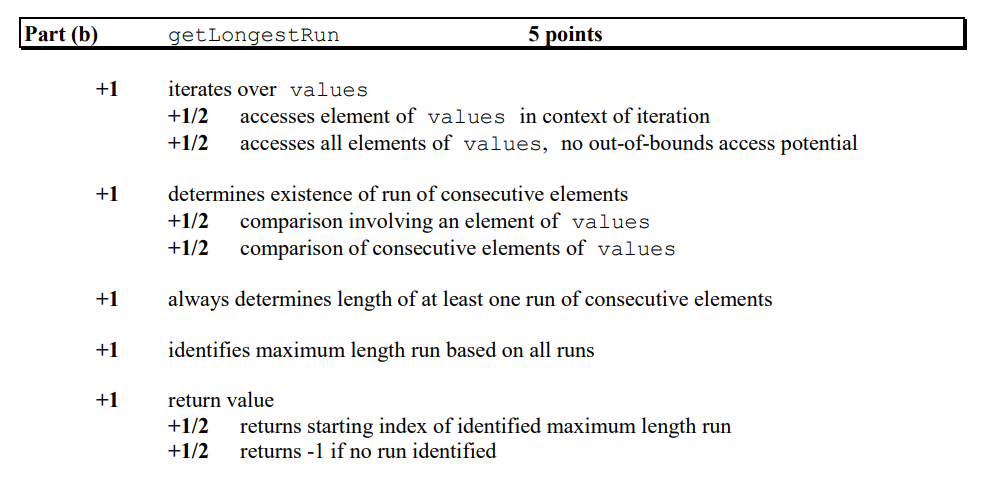
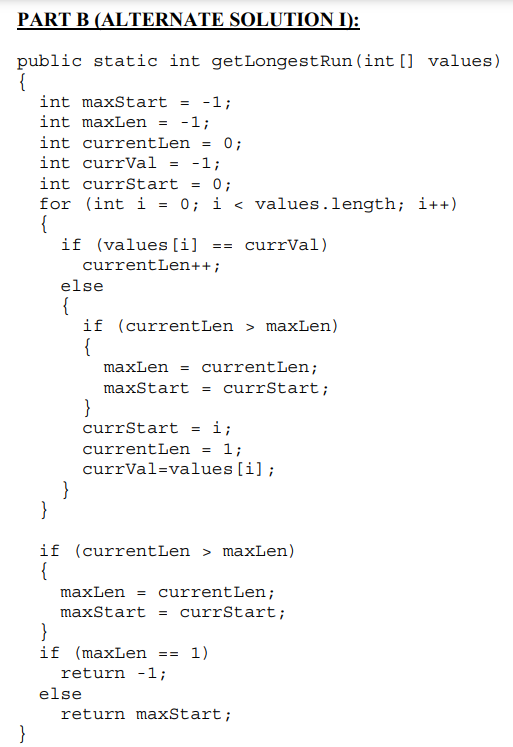
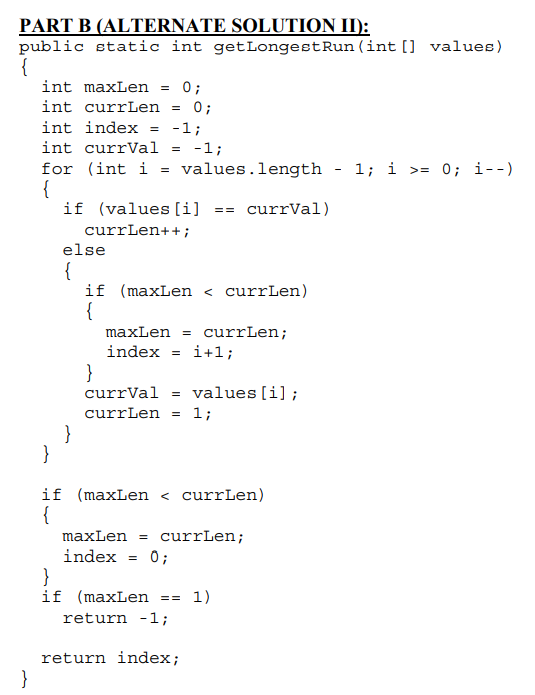
(다르게)
public class NumberCube {
// @return an integer value between 1 and 6, inclusive
public int toss() {
}
// (a)
public static int[] getCubeTosses(NumberCube cube, int numTosses) {
int[] myCube = new int[numTosses];
for(int i = 0; i < myCube.length; i++) {
myCube[i] = cube.toss();
}
return myCube;
}
// (b)
public static int getLongestRun(int[] values) {
int count = 0;
int maxLength = 0;
int maxIndex = -1;
for(int i = 0; i < values.length - 1; i++) {
if(values[i] == values[i+1]) {
count++;
if(count > maxLength) {
maxLength = count;
maxIndex = i - count + 1;
}
}
else {
count = 0;
}
}
return maxIndex;
}
}
728x90
반응형
'자바(Java) > 자바 AP' 카테고리의 다른 글
AP Computer Science A [FRQ] Practice (5) (0) | 2024.04.04 |
---|---|
AP Computer Science A [FRQ] Practice (4) (0) | 2024.04.04 |
AP Computer Science A [FRQ] Practice (2) (0) | 2024.04.04 |
AP Computer Science A [FRQ] Practice (1) (0) | 2024.04.04 |
APCSA Mock Test (0) | 2024.03.29 |
댓글